How to create Excel file in C#
The following C# code example shows how to use COM interop to create an Excel file. Before going to create new Excel file programmatically in C#, you must have Excel installed on your system for this code to run properly.
Excel Library
To access the object model from Visual C# .NET, you have to add the Microsoft Excel 15.0 Object Library to you project.
Create a new project in your Visual Studio and add a Command Button to your C# Form.
How to use COM Interop to Create an Excel Spreadsheet
Form the following pictures you can find how to add Excel reference library in your project.
Select Add Reference dialogue from Project menu of your Visual Studio.
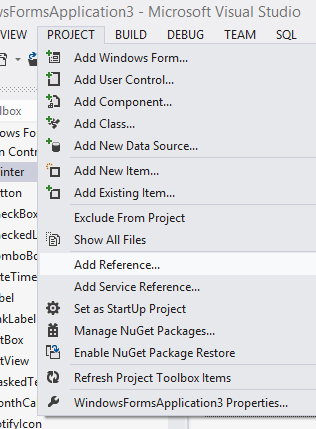
Select Microsoft Excel 15.0 Object Library of COM leftside menu and click OK button.
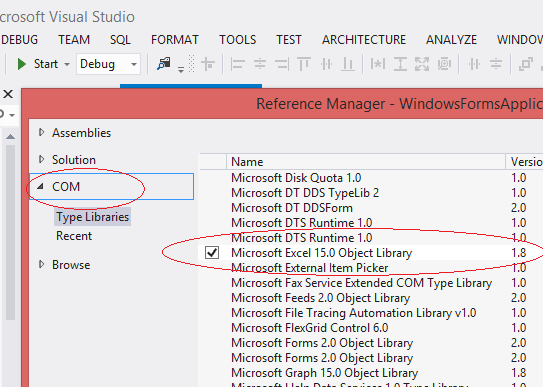
How to create an Excel Document Programmatically
First we have to initialize the Excel application Object.
Before creating new Excel Workbook, you should check whether Excel is installed in your system.
Then create new Workbook
After creating the new Workbook, next step is to write content to worksheet
In the above code we write the data in the Sheet1, If you want to write data in sheet 2 then you should code like this.
Save Excel file (SaveAs() method)t
After write the content to the cell, next step is to save the excel file in your system.
How to properly clean up Excel interop objects
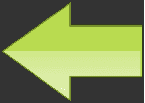
Interop marshaling governs how data is passed in method arguments and return values between managed and unmanaged memory during calls. Most data types have common representations in both managed and unmanaged memory. The interop marshaler handles these types for you. Other types can be ambiguous or not represented at all in managed memory.
It is important to note that every reference to an Excel COM object had to be set to null when you have finished with it, including Cells, Sheets, everything.
The Marshal class is in the System.Runtime.InteropServices namespace, so you should import the following namespace.
Creating an Excel Spreadsheet Programmatically
Copy and paste the following source code in your C# project file
Full Source C#Note : You have to add Microsoft.Office.Interop.Excel to your source code.
When you execute this program , it will create an excel file in you D:
- How to open an Excel file in C#
- How to read an Excel file using C#
- How to programmatically Add New Worksheets
- How to delete worksheet from an excel file
- How to format an Excel file using C#
- How to insert a picture in excel from C# App
- How to insert a background picture in excel
- How to create Excel Chart from C#
- How to export excel chart from C#
- How to excel chart in C# picturebox
- C# data validation input box in excel file
- Read and Import Excel File into DataSet or DataTable
- How to insert data to Excel file using OLEDB
- How to update data in Excel file using OLEDB
- How to export databse to excel file
- How to export DataGridView to excel file