AES Encryption In C#
AES (Advanced Encryption Standard) is a symmetric encryption algorithm that is widely used to provide confidentiality and integrity to data. It is a block cipher encryption algorithm, which means that it encrypts data in blocks of fixed length. AES was designed to replace the older encryption standard, DES (Data Encryption Standard), which was no longer considered secure enough for modern applications.
How does AES Encryption works?
AES encryption works by using a secret key to encrypt and decrypt data. The key length can be 128, 192, or 256 bits, and the longer the key, the more secure the encryption. AES encrypts data in a series of rounds, with each round consisting of several steps. During each round, the algorithm transforms the input data using a combination of substitution and permutation operations.
The encryption process starts by dividing the plaintext (the original data to be encrypted) into blocks of 128 bits. Each block is then processed by the AES algorithm using the secret key. The key is expanded into a series of round keys, which are used in each round to transform the input data. The algorithm applies a series of substitution and permutation operations to the input data using the round keys. This process is repeated for several rounds, with the number of rounds depending on the key length.
At the end of the encryption process, the output is a series of encrypted blocks that can only be decrypted using the same secret key. The decryption process is the reverse of the encryption process. The ciphertext (the encrypted data) is divided into blocks of 128 bits, and each block is processed by the AES algorithm using the same key that was used for encryption. The algorithm applies the inverse of the substitution and permutation operations used in the encryption process, using the same round keys. This process is repeated for several rounds, with the number of rounds depending on the key length.
Security of AES encryption
The security of AES encryption depends on the strength of the secret key used. AES is considered secure and is widely used in many applications, including finance, healthcare, and government agencies. However, as with any encryption algorithm, AES can be vulnerable to attacks if the key is weak or if the implementation is flawed. It is important to use a key with a sufficient length and to follow best practices for key management to ensure the security of AES encryption.
Implement AES Encryption in C#
C# provides built-in support for AES encryption through the System.Security.Cryptography namespace. You can use the AesManaged class to encrypt and decrypt data. Following is an example of how to encrypt a string using AES:
AES Encryption Block Size
The AES (Advanced Encryption Standard) encryption algorithm operates on blocks of data of a fixed size, which is determined by the block size parameter of the algorithm.
AES supports three block sizes: 128 bits (16 bytes), 192 bits (24 bytes), and 256 bits (32 bytes). Each block size has a corresponding key size (128-bit key for 128-bit blocks, 192-bit key for 192-bit blocks, and 256-bit key for 256-bit blocks). The larger the block size, the more secure the encryption, but also the slower the encryption process.
In .NET, the default block size for the AesManaged class is 128 bits (16 bytes), which is also the most commonly used block size for AES encryption. The block size can be set explicitly when creating an instance of the AesManaged class using the BlockSize property, but it is generally not recommended to change the default value unless you have specific requirements that require a different block size.
AES Encryption Key
The AES (Advanced Encryption Standard) encryption algorithm uses a symmetric key for encryption and decryption. This means that the same key is used for both encryption and decryption, and anyone who has access to the key can decrypt the encrypted data.
The AES key is a binary string of a specific size, which corresponds to the key size parameter of the algorithm. AES supports three key sizes: 128 bits (16 bytes), 192 bits (24 bytes), and 256 bits (32 bytes). The larger the key size, the more secure the encryption, but also the slower the encryption process.
In .NET, the AesManaged class is used for AES encryption and decryption, and it generates a new random key each time an instance of the class is created. The generated key can be obtained using the Key property of the class. Alternatively, a specific key can be used by setting the Key property to the desired value.
It is important to keep the AES key secure and protect it from unauthorized access, as anyone who has access to the key can decrypt the encrypted data. It is also recommended to use a strong and random key, and to change the key periodically to improve the security of the encrypted data.
AES encrypting and decrypting a String in C#
Explanation:
Above C# program demonstrates how to use the AES encryption algorithm to encrypt and decrypt a string.
First, the program generates a random AES key and initialization vector (IV) by creating a new instance of the AesManaged class. The original string is then converted to a byte array using UTF-8 encoding.
The Encrypt method is called to encrypt the byte array using the generated key and IV. This method creates a new instance of the AesManaged class and sets its key and IV to the ones passed as parameters. It then creates an encryptor using the key and IV and uses a CryptoStream to perform the encryption. Finally, it returns the encrypted bytes as an array.
The encrypted bytes are then converted to a base64-encoded string using the Convert.ToBase64String method and printed to the console.
Next, the Decrypt method is called to decrypt the base64-encoded string back to a byte array using the same key and IV used for encryption. This method works in a similar way to the Encrypt method, but creates a decryptor instead and uses a CryptoStream to perform the decryption. The decrypted byte array is then converted back to a string using UTF-8 encoding and printed to the console.
Initialization Vector (IV)
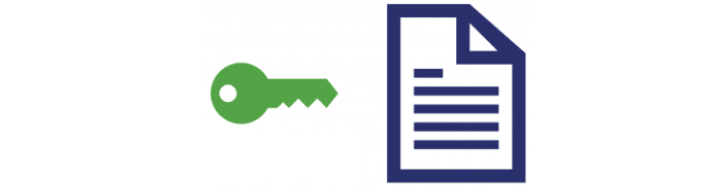
The Initialization Vector (IV) is a fixed-size binary string that is used as an additional input to the encryption algorithm along with the encryption key. The IV is used to ensure that the same plaintext input does not produce the same ciphertext output when encrypted with the same key.
The IV is usually the same length as the encryption algorithm's block size, which for AES is typically 128 bits (16 bytes). When encrypting data, the IV is combined with the encryption key to create a unique cryptographic context for each block of data being encrypted. The IV is usually generated randomly for each encryption operation, ensuring that the same plaintext input does not produce the same ciphertext output.
During decryption, the IV is used to recreate the same cryptographic context that was used during encryption, which is necessary to produce the correct plaintext output. The IV is usually sent along with the encrypted data or stored with it, as it is required to decrypt the data.
It is important to use a unique IV for each encryption operation, and to keep the IV secure along with the encryption key, as anyone who has access to the IV can use it to decrypt the encrypted data.
Encrypting and Decrypting a Byte array | C#
Following is an example program that encrypts and decrypts a byte array using AES encryption:
Explanation:
Above C# program demonstrates the use of the AES encryption algorithm to encrypt and decrypt a byte array using a randomly generated key and initialization vector (IV).
The program starts by creating a byte array called originalBytes containing some example data to be encrypted. Then, using the AesManaged class, it generates a random key and IV, which will be used to encrypt and decrypt the data.
The Encrypt function takes in the input byte array, key, and IV as parameters and returns the encrypted byte array. It creates a new instance of the AesManaged class and sets the key and IV properties. It then creates an encryptor using these values and writes the input byte array to a MemoryStream using a CryptoStream. The encrypted bytes are returned by converting the MemoryStream to a byte array.
The Decrypt function takes in the encrypted byte array, key, and IV as parameters and returns the decrypted byte array. It creates a new instance of the AesManaged class and sets the key and IV properties. It then creates a decryptor using these values and reads the encrypted byte array from a MemoryStream using a CryptoStream. The decrypted bytes are returned by converting the outputBytes byte array to a byte array.
The Main function calls the Encrypt function to encrypt the originalBytes array, and then prints the original and encrypted byte arrays. It then calls the Decrypt function with the encrypted bytes, and prints the decrypted byte array.
Is AES Encryption secure?
AES (Advanced Encryption Standard) encryption is widely considered to be secure, as it is a widely-used and well-studied encryption algorithm. AES has been subjected to extensive analysis by security researchers, cryptographers, and governments around the world. It has also been approved by the U.S. government for use in securing classified information.
That being said, no encryption algorithm can ever be 100% guaranteed to be secure, as vulnerabilities and attacks may be discovered in the future. It is important to use AES encryption correctly, such as choosing appropriate key sizes and using secure modes of operation, to maximize its security. It is also important to keep in mind that encryption is just one aspect of a comprehensive security strategy, and additional measures such as access controls, firewalls, and intrusion detection systems may also be necessary to protect sensitive data.