Dependency Injection in C#
Dependency Injection (DI) is a design pattern used in software development that helps manage the dependencies between objects or classes. The main idea of DI is to inject (provide) the required dependencies to a class or object from the outside, rather than letting the class create them internally.
In C#, Dependency Injection is typically implemented using a framework like Microsoft's Dependency Injection framework. Following an example of how Dependency Injection can be used in C#:
Implementation of Dependency Injection Pattern in C#
Suppose you have a class called UserService that needs to send emails to users when certain events occur. In order to send emails, UserService needs an instance of a MailService class. Without DI, UserService might create an instance of MailService internally, like the following:
However, this approach has some drawbacks. First, it creates a tight coupling between UserService and MailService. If you ever want to switch to a different email service, you will have to modify the UserService class. Second, it's hard to test UserService in isolation, since you can't easily substitute a different implementation of MailService.
With Dependency Injection, you can inject an instance of MailService into UserService from the outside. following code shows how that might look:
In the above version of the UserService class, the MailService instance is injected via the constructor, and it's stored in a private field called mailService. Note that instead of MailService, you are using an interface called IMailService. This is a common practice in Dependency Injection, since it makes it easy to substitute different implementations of IMailService as needed.
To use UserService, you need to create an instance of MailService, and then pass it to the UserService constructor:
With the Dependency Injection, you can hold UserService and MailService as separate stored objects. Thus this module becomes compatible with the needs of easier maintenance and testing. It is a piece of cake that you can change the implementation to the real service, and you can also do that with the mock one for the test.
Tight Coupling and Loose Coupling
In software design, coupling refers to the degree of interdependence between different modules or components of a software system. Tight coupling means that modules or components are highly interdependent, while loose coupling means that they are less interdependent.
Tight coupling can make a software system inflexible and difficult to maintain, since changes in one module may require changes in many other modules. On the other hand, loose coupling can make a system more flexible and easier to maintain, since changes in one module may have less of an impact on other modules.
Following are some examples to illustrate the difference between tight coupling and loose coupling:
Tight coupling:
A class that creates an instance of another class internally and calls its methods directly, like the following:
In the above example, the OrderProcessor class is tightly coupled to the PaymentProcessor class, since it creates an instance of PaymentProcessor internally and calls its Charge method directly. This makes it difficult to substitute a different payment processor implementation or test the OrderProcessor class in isolation.
Loose coupling:
A class that depends on an interface instead of a concrete implementation, and the implementation is passed to the class externally, like the following:
In the above example, the OrderProcessor class is loosely coupled to the payment processor implementation, since it depends on the IPaymentProcessor interface instead of a concrete implementation. The implementation is passed to the OrderProcessor class via its constructor, so you can easily substitute a different implementation or use a mock implementation for testing purposes.
Types of Dependency Injection (DI)
In C#, there are three common types of Dependency Injection (DI) design patterns:
- Constructor Injection
- Property Injection
- Method Injection
Constructor Injection:
Constructor Injection is the most commonly used DI pattern in C#. In Constructor Injection, dependencies are passed to a class through its constructor.
In the above example, the MyService class takes an instance of IDependency as a parameter in its constructor. The dependency is then stored in a private field for later use.
Property Injection:
Property Injection is another type of DI pattern in C#. In Property Injection, dependencies are passed to a class through its public properties.
In the above example, the MyService class has a public property called Dependency of type IDependency. The dependency is set by an external entity, typically a DI container, after an instance of MyService is created.
Method Injection:
Method Injection is the least common DI pattern in C#. In Method Injection, dependencies are passed to a class through its public methods.
In the above example, the MyService class has a public method called DoSomething that takes an instance of IDependency as a parameter. The dependency is passed to the method by an external entity, typically a DI container.
It's worth noting that Constructor Injection is generally considered the most flexible and maintainable DI pattern, since it allows dependencies to be provided in a consistent manner and makes them immediately available for use. Property Injection and Method Injection can be useful in certain scenarios, but they can also make code more difficult to reason about and test, since dependencies may not be immediately available or may change over time.
Constructor Injection in C#
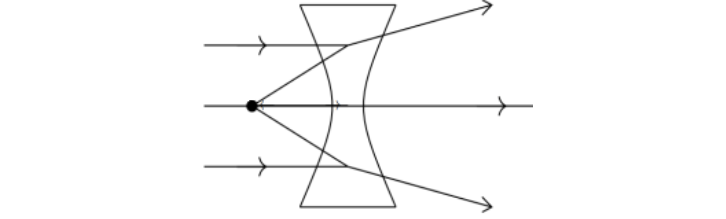
Constructor injection is a type of dependency injection, which is a design pattern used to invert the control of object creation and management. Instead of having an object create and manage its own dependencies, the dependencies are injected into the object from the outside.
Constructor injection has a number of benefits, including:
- It makes the code more testable, because dependencies can be easily mocked or substituted for testing purposes.
- It simplifies object creation, because the object's dependencies are clearly defined in its constructor.
- It makes the code more modular, because objects can be easily composed from smaller, reusable dependencies.
Working example of C# Constructor Injection:
In the above example, EmailService has a constructor that takes two dependencies: an ILogger and an IConfiguration. These dependencies are injected into the constructor when an instance of EmailService is created.
In the Main method, create instances of ConsoleLogger, AppConfigConfiguration, and EmailService, passing in the dependencies to the constructor of EmailService. Then call the SendEmail method on EmailService and output the result to the console.
These dependencies could be substituted for other implementations of ILogger and IConfiguration as needed.
Advantages of Dependency Injection
Dependency Injection (DI) is a design pattern used in software engineering to help reduce coupling between different modules of an application. Here are some advantages of Dependency Injection:
- Loose coupling: Dependency Injection promotes loose coupling between the different components of an application. It makes it easier to modify, test and maintain these components in isolation.
- Testability: One of the key benefits of DI is that it makes unit testing easier. By injecting dependencies into a component, it is possible to replace real dependencies with mock objects or test doubles. This allows developers to test components in isolation without having to worry about the behavior of other components.
- Reusability: DI promotes the reuse of components across different parts of an application. Since components are not tightly coupled, they can be reused in different contexts, which leads to a more modular and flexible codebase.
- Encapsulation: DI can help encapsulate the creation of dependencies in a single place, making it easier to manage them. This can also help to reduce the number of dependencies that need to be explicitly declared and passed around.
- Flexibility: The DI method allows the DI to be kept the same way by just being injected in allowed the behavior of the component to be changed. This softens the code and makes it more robust and easy to adjust to varying requirements.
Conclusion:
Dependency Injection can lead to a more maintainable, testable, and flexible codebase, which is easier to modify and extend over time.