Design Patterns In C#
Design patterns are reusable solutions to common problems that arise during software development. They provide a set of best practices and guidelines for creating software that is maintainable, scalable, and efficient. There are many design patterns that can be used in C#, and they are generally classified into three categories:
- Creational Patterns
- Structural Patterns
- Behavioral Patterns
Creational Patterns:
Creational patterns are used to create objects in a way that is flexible and efficient. These patterns focus on abstracting the process of object creation and hiding the implementation details from the client code. The most common creational patterns in C# are:
- Singleton pattern: This pattern ensures that only one instance of a class is created and provides global access to it.
- Factory pattern: This pattern provides an interface for creating objects, but lets subclasses decide which classes to instantiate.
- Abstract Factory pattern: This pattern provides an interface for creating families of related or dependent objects without specifying their concrete classes.
- Builder pattern: This pattern separates the construction of a complex object from its representation, allowing the same construction process to create different representations.
- Prototype pattern: This pattern allows new objects to be created by cloning existing objects, instead of creating new ones from scratch.
Structural Patterns
Structural patterns focus on the composition of classes and objects to form larger structures. They help to simplify the design of complex systems by identifying relationships between objects and defining interfaces between them. The most common structural patterns in C# are:
- Adapter pattern: This pattern allows incompatible classes to work together by converting the interface of one class to that of another.
- Bridge pattern: This pattern separates an abstraction from its implementation, allowing them to vary independently.
- Composite pattern: This pattern allows a group of objects to be treated as a single object, with a common interface.
- Decorator pattern: This pattern adds new functionality to an object by wrapping it in another object that adds the required behavior.
- Facade pattern: This pattern provides a simplified interface to a complex system, hiding its complexity from the client code.
Behavioral Patterns:
Behavioral patterns focus on the communication between objects and the distribution of responsibilities among them. They help to define the behavior of an object or a group of objects and improve the flexibility of the system. The most common behavioral patterns in C# are:
- Observer pattern: This pattern defines a one-to-many dependency between objects, so that when one object changes state, all its dependents are notified and updated automatically.
- Command pattern: This pattern encapsulates a request as an object, allowing it to be queued, logged, and undone.
- Template Method pattern: This pattern defines the skeleton of an algorithm in a superclass, allowing subclasses to override specific steps of the algorithm.
- State pattern: This pattern allows an object to change its behavior when its internal state changes.
- Strategy pattern: This pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable.
Design Patterns in With C# Real-time Example
Let's take the Observer pattern as an example. The Observer pattern is a behavioral pattern that allows one-to-many communication between objects. In this pattern, an object (called the subject) maintains a list of its dependents (called observers) and notifies them automatically of any state changes, so that they can update their state accordingly.
To illustrate the Observer pattern in C#, let's consider the following scenario: You have a weather station that measures temperature, humidity, and pressure, and you want to display this information on a dashboard. You can use the Observer pattern to update the dashboard automatically whenever the weather station measurements change.
Following is the C# code for this example:
Above C# example program demonstrates the Observer design pattern, which is used when there is a one-to-many relationship between objects such that when one object changes state, all its dependents are notified and updated automatically.
In the above program, the WeatherStation class is the subject that notifies its observers about changes in temperature, humidity, and pressure. It implements the IWeatherStation interface, which defines methods for adding, removing, and notifying observers.
The Dashboard class is an observer that displays the temperature, humidity, and pressure on a dashboard. It implements the IWeatherObserver interface, which defines the update method that gets called by the subject to update its state.
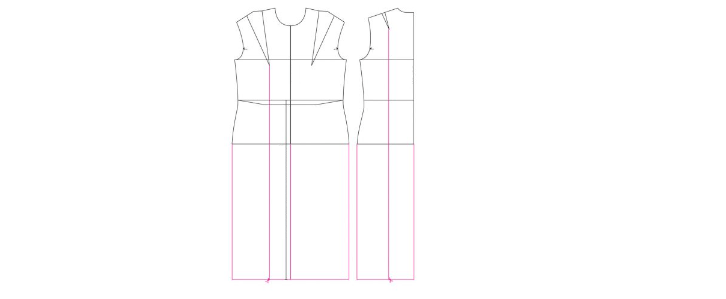
In the Main method, an instance of the WeatherStation class and an instance of the Dashboard class are created. The dashboard object is added as an observer to the weatherStation object using the AddObserver method. Then, the weather station's SetMeasurements method is called three times with different values to simulate changes in the weather conditions. Each time the SetMeasurements method is called, it updates the weather station's internal state and calls the NotifyObservers method, which in turn calls the Update method on each observer.
After the third call to SetMeasurements, the dashboard object is removed as an observer using the RemoveObserver method. Finally, the program waits for a key to be pressed before terminating.