What is Entity Framework?
Entity Framework is an object-relational mapping (ORM) framework for .NET applications. It enables developers to work with data in the form of domain-specific objects and properties, without having to write low-level data access code or interact directly with the database. Entity Framework can simplify data access code by allowing developers to work with domain-specific objects and properties, rather than writing complex SQL queries. It can also handle many common database-related tasks, such as generating SQL statements, tracking changes, and handling concurrency conflicts.
Features of Entity Framework
Following are some of the key features of Entity Framework:
- Object-Relational Mapping: Entity Framework provides a powerful and flexible way to map objects to relational database tables. This allows developers to work with data in an object-oriented way without having to worry about the underlying database schema.
- LINQ Support: Entity Framework provides support for Language Integrated Query (LINQ) which allows developers to write complex queries in a more natural and expressive way using C# or Visual Basic .NET (VB.NET) syntax.
- Code-First Development: Entity Framework also supports Code-First development, which allows developers to write code and define classes first and then Entity Framework generates the database schema based on those classes.
- Database Migrations: Entity Framework also supports database migrations, which allows developers to easily update their database schema as their application evolves.
- Change Tracking: Entity Framework tracks changes made to objects and automatically generates the SQL statements required to persist those changes to the database.
- Lazy Loading: Entity Framework supports lazy loading, which allows developers to load related entities only when needed, reducing the amount of data retrieved from the database.
- Transactions: Entity Framework also provides transaction support, allowing developers to perform multiple operations as a single transaction, ensuring data consistency and integrity.
- Connection Management: Entity Framework handles connection management automatically, allowing developers to focus on writing code without worrying about managing database connections.
In which scenarios Entity Framework is using in C#
Entity Framework (EF) is commonly used in scenarios where developers want to:
Build applications that interact with databases:
With Entity Framework, developers can write code that interacts with the database using C# objects instead of SQL statements. This makes it easier to build applications that need to store and retrieve data.
Abstract database complexity:
Entity Framework allows developers to work with databases at a higher level of abstraction, hiding the complexity of database operations such as creating tables, defining relationships between tables, and executing SQL statements.
Improve productivity:
Entity Framework permits developers to stay focused on developing their application logic and not getting involved with the details regarding repetitive code regarding database access. EF as a compact arsenal that serves for the implementation of the algorithms, the models’ validation and the simplification of the databases’ work.
Increase maintainability:
Entity Framework makes it easier to maintain code because developers can work with C# objects instead of SQL statements. This makes it easier to change the database schema or modify the database access logic without affecting the rest of the application code.
Entity Framework Architecture
The architecture of Entity Framework can be divided into four main components:
- Entity Data Model (EDM): The EDM represents the conceptual data model used by the application. It defines the entities, relationships between entities, and their properties. The EDM is defined using an Entity Data Model designer tool or by code in the case of the Code-First approach.
- Object Services: Object Services provides the runtime environment for working with objects in the EDM. It includes a set of services that handle operations such as querying, updating, and caching data. Object Services generates SQL statements based on LINQ queries or method calls on the objects in the EDM.
- Entity Client Data Provider:The Entity Client Data Provider is responsible for translating the SQL statements generated by Object Services into commands that can be executed against the database. It also handles connections, transactions, and data types.
- Data Store:The Data Store represents the database that is used to store the application's data. The Data Store can be any database that is supported by EF, such as SQL Server, Oracle, or MySQL.
When an application using EF is executed, the Entity Client Data Provider connects to the Data Store and executes the SQL commands generated by Object Services. The results are returned as objects in the EDM, which are then used by the application. When data is updated or inserted into the database, Object Services generates the appropriate SQL commands and passes them to the Entity Client Data Provider.
EF also provides a set of tools for working with the database schema, such as generating the database schema from the EDM or updating the database schema based on changes in the EDM.
Entity Framework Development Approaches
There are two main approaches to developing applications with Entity Framework (EF) in C#:
- Code-First
- Database-First
- Model First Approach
Code-First approach:
In this approach, developers start by creating the C# classes that define the entities in their application (e.g., customers, orders, products). These classes are then used to generate the database schema and the corresponding database tables. The Code-First approach allows for a more object-oriented approach to data modeling and is often used in greenfield applications where the database schema is not yet defined.
Database-First approach:
In this approach, developers start by creating the database schema using a tool like SQL Server Management Studio or another database design tool. EF is then used to generate the corresponding C# classes and code that will be used to interact with the database. The Database-First approach is often used in applications that need to work with an existing database schema.
Both approaches have their advantages and disadvantages, and the choice of which one to use depends on the specific needs of the application. For example, the Code-First approach allows for more flexibility in data modeling and is better suited for applications that require frequent changes to the database schema. On the other hand, the Database-First approach may be more appropriate for applications that need to work with a pre-existing database schema.
In addition to these two approaches, there is also a Hybrid approach that combines elements of both Code-First and Database-First. In this approach, developers can start with an existing database schema and use EF to generate C# classes and code that are then manually modified to match the specific needs of the application.
Model First Approach
The Model-First approach is another way to develop applications with Entity Framework (EF) in C#. This approach involves designing the data model using an Entity Data Model (EDM) designer tool, such as the one included in Visual Studio.
With the Model-First approach, developers start by designing the data model using the EDM designer tool. This involves creating entities, defining their relationships, and specifying properties and constraints. Once the data model is defined, the tool generates the corresponding database schema and the code that will be used to interact with the database.
The generated code includes C# classes that represent the entities in the data model and a set of database access methods that are used to retrieve, create, update, and delete data. These classes and methods can be customized as needed to implement the specific requirements of the application.
The Model-First approach is often used in scenarios where the data model is the primary focus of the application, and there is less emphasis on the application logic. For example, it may be used in data-centric applications such as reporting or analytics tools.
Like the Code-First and Database-First approaches, the Model-First approach has its advantages and disadvantages. It can be useful for quickly prototyping data models and generating corresponding database schemas and code. However, it may be less flexible than the other approaches when it comes to customizing the generated code, and it may not be as suitable for applications that require a more complex data access layer or application logic.
Basic Workflow in Entity Framework
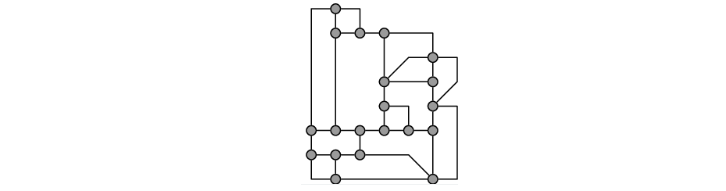
The basic workflow of Entity Framework (EF) can be divided into several steps:
- Define the Entity Data Model (EDM):The first step is to define the EDM, which represents the conceptual data model used by the application. The EDM is defined using an Entity Data Model designer tool or by code in the case of the Code-First approach. The EDM includes the entities, relationships between entities, and their properties.
- Create DbContext class: The next step is to create a DbContext class, which represents a session with the database and provides access to the entities in the EDM. The DbContext class is responsible for managing database connections, executing queries, and tracking changes to entities.
- Querying data: The next step is to query data from the database using LINQ or method syntax. EF translates the queries into SQL statements that are executed against the database, and the results are returned as objects in the EDM.
- Modifying data:To modify data in the database, the application modifies the properties of the entities in the EDM and calls the SaveChanges() method on the DbContext. EF then generates the appropriate SQL statements to update or insert the data in the database.
- Handling concurrency:EF provides a mechanism for handling concurrency, which occurs when multiple users try to modify the same data at the same time. EF can detect conflicts and provide options for resolving them.
- Handling transactions:EF supports transactions, which are used to group multiple database operations into a single unit of work. Transactions ensure that either all operations are completed successfully or none of them are.
- Updating the database schema:EF provides a set of tools for updating the database schema based on changes to the EDM. These tools can generate SQL scripts to update the database schema, or they can automatically apply the changes to the database.
By providing a high-level abstraction over the database, EF makes it easier to work with databases in C# and allows developers to focus on the application logic rather than the details of database access.
How to get started with Entity Framework (EF)
Install the Entity Framework package:
The first step is to install the EF package. If you are using Visual Studio, you can do this by opening the NuGet Package Manager and searching for "Entity Framework". Alternatively, you can install the package using the Package Manager Console by running the command: Install-Package EntityFramework
Create a new project:
Once you have installed the EF package, create a new project in Visual Studio. You can choose from several project templates, such as Console Application, ASP.NET MVC, or WPF Application.
Define the Entity Data Model (EDM):
The next step is to define the EDM, which represents the conceptual data model used by the application. You can define the EDM using an Entity Data Model designer tool or by code in the case of the Code-First approach.
Create DbContext class:
After defining the EDM, create a DbContext class, which represents a session with the database and provides access to the entities in the EDM. The DbContext class is responsible for managing database connections, executing queries, and tracking changes to entities.
Querying data:
To query data from the database, use LINQ or method syntax. EF translates the queries into SQL statements that are executed against the database, and the results are returned as objects in the EDM.
Modifying data:
To modify data in the database, modify the properties of the entities in the EDM and call the SaveChanges() method on the DbContext. EF then generates the appropriate SQL statements to update or insert the data in the database.
Handling concurrency:
EF provides a mechanism for handling concurrency, which occurs when multiple users try to modify the same data at the same time. EF can detect conflicts and provide options for resolving them.
Handling transactions:
EF supports transactions, which are used to group multiple database operations into a single unit of work. Transactions ensure that either all operations are completed successfully or none of them are.
Updating the database schema:
EF provides a set of tools for updating the database schema based on changes to the EDM. These tools can generate SQL scripts to update the database schema, or they can automatically apply the changes to the database.
Conclusion:
Entity Framework provides a rich set of features that makes working with data in .NET applications much easier and more productive. It simplifies the data access code in C# applications and provides a powerful and flexible way to work with data. It saves time and effort in creating and maintaining data access code, improves performance, and reduces the potential for errors. It is commonly used in scenarios such as building web applications, desktop applications, and enterprise applications that need to interact with databases.