Encapsulation in C# with Examples
Encapsulation in C# is a fundamental concept in object-oriented programming that refers to the idea of wrapping data and behavior within a single unit, which is called a class. Encapsulation provides a mechanism for protecting the internal state of an object from external interference and prevents unauthorized access to its data.
In C#, encapsulation is achieved by defining the class's fields as private or protected, which means that they are not directly accessible from outside the class. Access to the class's fields is provided through methods, which are called "accessors" or "getters" and "mutators" or "setters". These methods are declared as public and provide controlled access to the class's data.
Encapsulation in C# provides several benefits, including:
- Data hiding: Encapsulation allows the data of a class to be hidden from external code, which can help prevent unintended modification or corruption of data.
- Abstraction: By encapsulating the implementation details of a class, the interface to the class can be simplified and abstracted, making it easier to use and maintain.
- Modularity: Encapsulation allows for the creation of modular code, where each class encapsulates a specific set of functionality, which can be reused in other parts of the codebase.
Encapsulation is a key principle of object-oriented programming and is essential for creating robust, maintainable, and reusable code in C#.
Why is encapsulation important in C# programming?
Encapsulation is an essential concept in C# programming, and there are several reasons why it is so important:
Data protection:
Encapsulation allows data to be hidden and protected from external access, ensuring that data cannot be accidentally or intentionally modified in unexpected ways. This helps maintain the integrity of data, reducing the likelihood of errors and improving the reliability of the code.
Code reuse:
Encapsulation makes it easier to reuse code, as it allows functionality to be packaged into discrete, reusable components. This reduces the amount of code that needs to be written, tested, and maintained, leading to faster development cycles and increased productivity.
Abstraction:
Encapsulation promotes abstraction, which allows complex systems to be broken down into smaller, more manageable pieces. This can make code easier to understand, debug, and maintain, as the complexity of the system is hidden behind well-defined interfaces.
Maintainability:
Encapsulation improves code maintainability by reducing dependencies between components. This makes it easier to change one part of the system without affecting other parts, reducing the likelihood of bugs and improving the overall stability of the codebase.
How can you achieve encapsulation in C# using access modifiers
Encapsulation in C# can be achieved using access modifiers to control the visibility of class members (fields, methods, properties, and events) from outside the class.
Access modifiers specify the level of access that other classes or objects have to a particular member. The four access modifiers in C# are:
- public: The public access modifier allows a member to be accessed from any code in the project, including other classes and assemblies.
- private: The private access modifier restricts a member's access to only the class in which it is defined.
- protected: The protected access modifier restricts a member's access to the class in which it is defined and its derived classes.
- internal: The internal access modifier allows a member to be accessed within the same assembly but not from outside the assembly.
To achieve encapsulation in C#, the class's fields should be declared as private or protected, and their values should be accessed through public methods or properties. These methods or properties can have their own access modifiers to control their visibility, but they should always provide controlled access to the internal state of the object.
Implimenation of encapsulation in C#
Encapsulation is implemented in C# by defining classes and controlling the access to their members, such as fields and methods. Following are the steps to implement encapsulation in C#:
Define a class:
To implement encapsulation, you need to create a class that will encapsulate the data and behavior of an object.
Declare fields:
Declare fields (data members) that hold the state of the object. To implement encapsulation, declare these fields with private or protected access modifiers to limit direct access from outside the class.
Create public methods:
Create public methods (member functions) that provide controlled access to the private fields. These methods are called "accessors" or "getters" and "mutators" or "setters" and are declared with the public access modifier.
Use properties:
In C#, you can use properties to encapsulate fields and provide access to their values. Properties are special methods that allow you to get or set the value of a field while controlling access to it.
In the above example, the Person class has private fields for name and age. These fields are accessed through public properties, Name and Age, respectively. Properties enable you to decide how the private fields will be accessed, the objects that can modify their values, and validation.
Purpose of getters and setters in C# encapsulation
Getters and setters are methods used in C# to provide controlled access to private fields or variables of a class. They are a key part of encapsulation, which is the practice of hiding the internal details of an object and providing access to its data only through well-defined public interfaces.
Getters and setters are used to read and write the values of private fields or variables of a class. They allow you to control how a field is accessed, set restrictions on what values can be assigned, and provide validation logic.
In the above example, the Name and Age properties provide controlled access to the private name and age fields, respectively. The get method of each property retrieves the value of the private field, and the set method assigns a new value to the field after performing some validation logic.
The Age property, for example, checks if the assigned value is within a valid age range (0 to 120), and throws an exception if the value is invalid.
Real time example of encapsulation in C#
In the above example, the class Person has two private fields name and age, and two public properties Name and Age that provide controlled access to these fields.
The private fields name and age are hidden from the rest of the program and can only be accessed within the class. This means that their values cannot be changed by other parts of the program directly.
The public properties Name and Age provide controlled access to these private fields. They are exposed to the rest of the program and can be used to retrieve or modify the values of name and age.
In the above code, create an instance of the Person class and set its Name and Age properties. The Console.WriteLine() statements then retrieve these values using the same properties.
Note that you did not access the private fields name and age directly, but instead used the public properties Name and Age. This demonstrates how encapsulation allows you to control the access to a class's internal data, making it easier to maintain and secure.
Advantages of encapsulation in C#
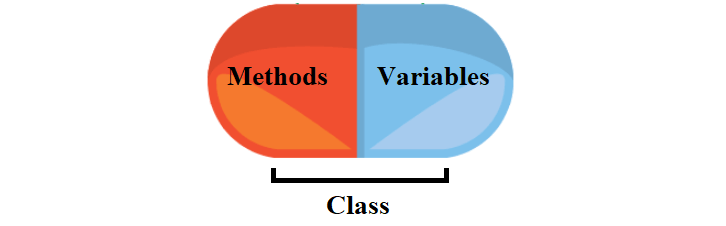
Encapsulation is a fundamental principle of object-oriented programming and has several advantages in C# programming:
- Data hiding: Encapsulation allows you to hide the internal details of a class and expose only what is necessary. This prevents other parts of the code from accessing or modifying the object's data directly, which can help prevent errors and make it easier to maintain the code.
- Improved security: By hiding the implementation details of a class, encapsulation makes it more difficult for malicious code to exploit vulnerabilities in the code.
- Abstraction: Encapsulation provides a way to abstract away the complexity of a class's implementation and expose only its essential features to the rest of the program. This simplifies the program's design and makes it easier to work with.
- Modularization: Encapsulation helps to divide a program into smaller, more manageable modules. By encapsulating a class's implementation details, it becomes easier to modify and maintain the class without affecting the rest of the program.
- Code reuse: Encapsulation makes it possible to reuse code in different parts of a program without having to modify it. A class's implementation details are hidden, and its public interface remains the same, allowing it to be used in different contexts.
How does encapsulation improve code maintainability in C#
Encapsulation improves code maintainability in C# by hiding the internal details of a class and providing access to its data only through well-defined public interfaces. This means that changes to the internal implementation of a class do not affect the way other parts of the program interact with it, as long as the public interface remains the same.
Following are some ways encapsulation improves code maintainability in C#:
Reduces dependencies:
Encapsulation helps reduce dependencies between different parts of the code by hiding the implementation details of a class. This means that if the implementation of a class changes, the rest of the program does not need to be modified.
Eases debugging:
Encapsulation can make debugging easier by localizing errors within a class. When a class is encapsulated, the rest of the program cannot access its internal data directly. This means that errors in the data can be traced back to the class that caused them.
Facilitates testing:
Encapsulation can make testing easier by making it easier to isolate the behavior of a class from the rest of the program. This means that the behavior of a class can be tested in isolation, without worrying about how it interacts with other parts of the program.
Simplifies maintenance:
Encapsulation can simplify maintenance by making it easier to modify the internal implementation of a class without affecting the rest of the program. This means that classes can be modified and updated without worrying about how they are used elsewhere in the program.
How does encapsulation enhance security in C# programming
Encapsulation enhances security in C# programming by hiding the internal implementation details of a class and providing access to its data only through well-defined public interfaces. This prevents unauthorized access and modification of the object's data by other parts of the program.
Following are some ways encapsulation enhances security in C# programming:
- Data hiding: Encapsulation hides the implementation details of a class and exposes only what is necessary through its public interface. This means that the rest of the program cannot access or modify the object's data directly, which helps prevent unauthorized access and modification.
- Controlled access: Encapsulation allows you to control the way a class's data is accessed and modified through its public interface. This means that you can enforce rules and constraints on how the data is used, preventing unauthorized access and modification.
- Validation and error checking: Encapsulation allows you to implement validation and error checking on a class's data. This means that you can prevent unauthorized or invalid data from being assigned to the object's data, further enhancing security.
Difference between encapsulation and abstraction in C#
Encapsulation and abstraction are both fundamental concepts in object-oriented programming, but they serve different purposes.
Encapsulation
Encapsulation is a mechanism for wrapping data and behavior into a single unit, such as a class, and controlling access to the internal state of an object. Encapsulation helps to protect the internal state of an object from external interference and prevents unauthorized access to its data. In C#, encapsulation is achieved by defining the class's fields as private or protected, and providing controlled access to the fields through public methods or properties.
Abstraction
Abstraction, on the other hand, is a mechanism for hiding the implementation details of an object and exposing only the essential features and functionality of the object to the outside world. Abstraction is achieved by defining abstract classes and interfaces, which provide a way to define a common set of behaviors for a group of related objects without specifying their implementation details. In C#, abstraction is achieved using abstract classes and interfaces, which define a set of methods that must be implemented by derived classes.
Conclusion:
Encapsulation is a key principle of object-oriented programming and is essential for creating robust, maintainable, and reusable code in C#.