Introduction to C# Unit Test for beginners
In C#, Unit Test is a type of automated testing that verifies the behavior of an individual unit of code, such as a method or function, in isolation from the rest of the application. The purpose of unit testing is to ensure that each unit of code performs correctly and as expected, and to detect any issues or bugs in the code early in the development process.
By writing unit tests for each unit of code in an application, developers can be confident that changes made to the code will not break existing functionality, and that the application as a whole will work as intended. Unit tests are typically written using a testing framework such as NUnit, MSTest, or xUnit, which provide a set of tools and methods for organizing and running tests, as well as reporting on the results.
Advantages of unit test in C#
There are several advantages of unit testing in C#:
- Catching errors early: By testing each unit of code in isolation, developers can catch errors and bugs early in the development process, when they are easier and cheaper to fix.
- Simplifying debugging: When a unit test fails, it provides a clear indication of which unit of code is causing the problem, which can help simplify the debugging process.
- Improving code quality: Unit tests encourage developers to write modular, reusable, and testable code, which can lead to better overall code quality.
- Facilitating refactoring: By ensuring that each unit of code is thoroughly tested, developers can be more confident when refactoring the code, since they can verify that the behavior of the code has not been changed.
- Enabling continuous integration and delivery: Unit tests can be integrated into automated build and deployment processes, which can help ensure that new changes to the code do not introduce new errors or regressions.
- Boosting team productivity: By automating the testing process and catching errors early, unit tests can help developers work more efficiently and reduce the time spent on manual testing and bug fixing.
A simple Unit Test program in C#
Suppose you have a class named "Calculator" that contains a method named "Add" that adds two numbers together. following is the code for the "Calculator" class:
To write a unit test for the "Add" method, you would create a separate test class with a method that tests the behavior of the "Add" method:
Key components of a unit test in C#
Following is an explanation of the key components of a unit test in C#:
TestClass:
A TestClass is a class that contains one or more unit tests. It is decorated with a [TestClass] attribute to indicate to the testing framework that it contains tests.
TestMethod:
A TestMethod is a method within a TestClass that contains the code for a unit test. It is decorated with a [TestMethod] attribute to indicate to the testing framework that it is a test method.
Arrange:
The Arrange phase of a unit test is where you set up the necessary preconditions for the test. This typically involves creating any necessary objects, setting up any required dependencies, and setting any initial values.
Act:
The Act phase of a unit test is where you execute the code that you want to test. This typically involves calling a method or function on an object, passing in any required arguments, and capturing any return values.
Assert:
The Assert phase of a unit test is where you verify that the actual results of the code match the expected results. This typically involves using assertion methods provided by the testing framework to compare actual values to expected values.
By combining these elements together in a structured way, you can write effective and reliable unit tests for your C# code.
NUnit | testing framework for the .NET
NUnit is a popular open-source unit testing framework for the .NET ecosystem. It allows developers to write automated tests in C# or other .NET languages to ensure that their code works as expected. NUnit provides a wide range of assertions and test runners that can help developers to write comprehensive and effective unit tests.
NUnit is widely used by developers who practice Test-Driven Development (TDD) or Behavior-Driven Development (BDD) to write tests before writing code. This helps to ensure that code is written with a focus on meeting specific requirements and that all code is thoroughly tested before being released to production.
NUnit is easy to install and use, and integrates well with many popular development environments, including Visual Studio and JetBrains Rider. It also provides support for parallel test execution, test categorization, and parameterized tests, making it a versatile tool for developers to use in their unit testing workflows.
C# program with a unit test using NUnit framework
Following is an example C# program with a unit test using NUnit framework:
And here's the corresponding NUnit test:
In the above example, the C# program contains two simple math functions, Add() and Subtract(). The NUnit test includes two unit tests: TestAdd() and TestSubtract(), which use the Assert.AreEqual() method to check the expected results of each function.
To run this test and view the results, you can use a test runner like NUnit Console Runner or NUnit GUI Runner. When the tests are run, the output will show which tests passed and which ones failed, along with any error messages or stack traces that were generated during the test run.
Unit testing frameworks for .NET
There are several popular unit testing frameworks for .NET, including:
- NUnit: NUnit is a popular open-source testing framework for .NET, which provides a range of tools and attributes for organizing, running, and reporting on tests.
- MSTest: MSTest is Microsoft's built-in testing framework for .NET, which is included with Visual Studio. It provides a range of tools for running and managing tests, and integrates well with other Microsoft tools and technologies.
- xUnit: xUnit is an open-source testing framework for .NET, which is designed to be lightweight, flexible, and extensible. It provides a range of tools for running and managing tests, and supports a wide range of testing scenarios.
- NUnitLite: NUnitLite is a lightweight version of NUnit, which is designed for use in mobile and embedded environments, where memory and processing power may be limited.
- MbUnit: MbUnit is an open-source testing framework for .NET, which provides a range of advanced features and tools, such as data-driven tests, parallel test execution, and fluent assertions.
- SpecFlow: SpecFlow is a testing framework for .NET, which is designed for Behavior-Driven Development (BDD). It allows developers to write tests in a natural language format, which can be easily understood by business stakeholders and non-technical team members.
The choice of unit testing framework will depend on your specific needs and requirements, as well as your development environment and toolchain.
Test Driven Design/Development
Test Driven Design (TDD), also known as Test Driven Development, is a software development methodology that emphasizes writing automated tests before writing the code that implements the features being tested. The basic idea behind TDD is to write a test that defines the desired functionality of a module or system, and then write the code necessary to pass that test.
The TDD process typically involves the following steps:
- Write a failing test: The developer writes an automated test that describes the desired functionality of a module or system, but which currently fails because the required code has not yet been implemented.
- Write the minimum code to pass the test: The developer writes the simplest possible code that will make the failing test pass. This code should be designed only to satisfy the current test, and should not include any unnecessary features or functionality.
- Refactor the code: Once the test is passing, the developer refactors the code to improve its design, remove duplication, and improve performance. This ensures that the code is maintainable and efficient over time.
- Repeat: The developer repeats this process for each new feature or piece of functionality, continually adding new tests and code, and refactoring as necessary.
The benefits of TDD include:
Improved code quality:
By writing tests before writing code, developers are forced to think carefully about the desired behavior of their code, which can lead to better-designed, more reliable software.
Faster feedback:
TDD provides immediate feedback on whether code changes are correct, which can help developers catch and fix errors more quickly.
Easier maintenance:
TDD encourages the use of clean, modular code, which is easier to maintain and extend over time.
Better documentation:
The tests themselves can serve as a form of documentation, explaining how the code is intended to behave and providing examples of usage.
Summary:
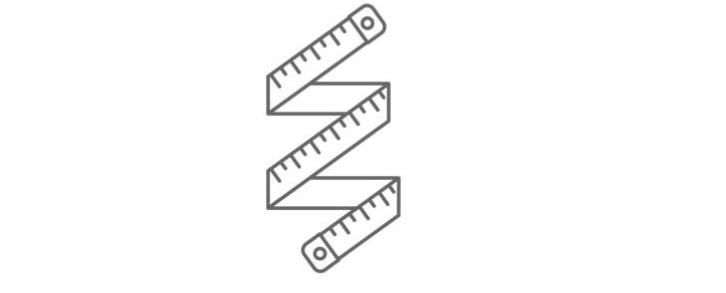
Unit testing is an important practice in software development that can help improve the quality of your code, reduce the number of bugs, and make it easier to maintain and refactor your code over time. By writing unit tests, you can verify that each piece of code in your application is working as expected, and catch any issues early on in the development process, before they become more difficult and expensive to fix. Additionally, writing unit tests can also help you to document your code and make it easier for others to understand how your code works. It can also make it easier to collaborate with other developers and ensure that your code works well with other parts of the application. Also, it is definitely worth considering as part of your software development process.