How to use C# List Class
The List class in C# is a fundamental component that represents a collection of objects with a strong type association.
List<T> Class- The parameter T is the type of elements in the list.
C# List Class offers the ability to access individual elements within the list through an index-based approach, facilitating efficient retrieval and manipulation of data. One of the key advantages of using the List class is its ability to store values of a specific type without requiring explicit casting to or from the general object type.
Add elements in List collection
Add Integer values in the List collectionCount list elements
To count the elements in a C# list, you can use the Count property provided by the List class. This property returns the total number of elements present in the list. Here's an example of how you can count the elements in a C# list:
In the bove example, we create a List<int> called "numbers" and initialize it with some integer values. The Count property is then used to retrieve the count of elements in the list, which is assigned to the variable "count". Finally, we display the count using the Console.WriteLine method.
Retrieve List elements
To retrieve elements from a C# List, you can use the indexing syntax or iteration. Here are examples of how you can retrieve elements from a List:
Using Indexing:In the above example, we create a List<string> called "colors" and initialize it with some fruit names. We retrieve individual elements by their index using square brackets and assign them to variables. We then display the retrieved elements using the Console.WriteLine method.
Using Iteration:In this example, we use a foreach loop to iterate over the List and retrieve each element. Within the loop, we can perform operations on each element or simply display it using the Console.WriteLine method.
Insert List elements

You can use insert(index,item) method to insert an in the specified index.
In the above code the color "violet" inserted in the index position 1.
How to sort a C# List
You can use the sort() method of C# List for ordering items in the List.
How to remove an item from List collection ?
Remove() can use to remove item from List collection.
How to check if an item exist in the List collection ?
You can use List.Contains() methods to check an item exists in the List
How to copy an Array to a List collection ?
How to Convert List to String in C#
You can convert a C# List to a string use the following method.
The output look like "Red,Blue,Green"
You can replace the seperator to any character instead of ","
Convert List to Array in C#
You can convert a C# List to an Array using toArray() method.
How to empty a list in C#?
Finally clear method remove all the items from List collection.
List Vs Array
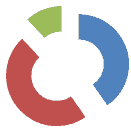
Arrays are memory-efficient . Lists are built on top of arrays. Because of this, Lists use more memory to store the same data. Array is useful when you know the data is fixed length , or unlikely to grow much. It is better when you will be doing lots of indexing into it, i.e. you know you will often want the third element, or the fifth, or whatever. On the other hand, if you don't know how many elements you'll have, use List. Also, definitely use a List any time you want to add/remove data, since resizing arrays is expensive.
If you are using foreach then the key difference is as follows:
Array:
- no object is allocated to manage the iteration
- bounds checking is removed
List via a variable known to be List :
- the iteration management variable is stack allocated
- bounds checking is performed
C# List to string
In C#, the ToString() method is used to obtain a string representation of an object. However, when it comes to List<T> in C#, calling ToString() on a list will not give you a meaningful representation of its contents. By default, List<T> inherits the ToString() implementation from the Object class, which returns the fully qualified name of the list's type.
If you want to get a string representation of the elements in a List<T>, you can use the string.Join() method to concatenate the elements into a single string with a specified delimiter.
How to get duplicate items from a list using LINQ
The GroupBy keyword groups the elements that are the same together, and the Where keyword filters out those that only appear once, leaving you with only the duplicates .
The following C# program shows the implementation of the above functionalities in List collection.
Last Updated: 20-Jun-2023