How to send email from C#
What is SMTP ?
SMTP (Simple Mail Transfer Protocol) is a part of the application layer of the TCP/IP protocol. It is an Internet standard for electronic mail (email) transmission. The default TCP port used by SMTP is 25 and the SMTP connections secured by SSL, known as SMTPS, uses the default to port 465.
SMTP Servers
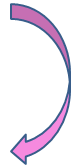
SMTP provides a set of protocol that simplify the communication of email messages between email servers. Most SMTP server names are written in the form "smtp.domain.com" or "mail.domain.com": for example, a Gmail account will refer to smtp.gmail.com. It is usually used with one of two other protocols, POP3 or IMAP, that let the user save messages in a server mailbox and download them periodically from the server.
SMTP Auhentication
SMTP Authentication, often abbreviated SMTP AUTH, is an extension of the SMTP (Simple Mail Transfer Protocol) whereby an SMTP client may log in using an authentication mechanism chosen among those supported by the SMTP servers.
How do I send mail using C#?
The Microsoft .NET framework provides two namespaces, System.Net and System.Net.Sockets for managed implementation of Internet protocols that applications can use to send or receive data over the Internet . SMTP protocol is using for sending email from C#. SMTP stands for Simple Mail Transfer Protocol . C# using System.Net.Mail namespace for sending email . We can instantiate SmtpClient class and assign the Host and Port . The default port using SMTP is 25 , but it may vary different Mail Servers .
Send Email using Gmail
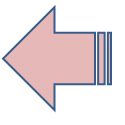
The following C# source code shows how to send an email from a Gmail address using SMTP server. The Gmail SMTP server name is smtp.gmail.com and the port using send mail is 587 and also using NetworkCredential for password based authentication.
Full Source C#You have to provide the necessary information like your gmail username and password etc.
- How to send email with attachment from C#
- How to send html email from C#
- How to send cdo email from C#
- How to find hostname of a computer
- How to find IP Adress of a computer
- How to read URL Content from webserver
- How to C# Socket programming
- C# Server Socket program
- C# Client Socket program
- C# Multi threaded socket programming
- C# Multi threaded Server Socket programming
- C# Multi threaded Client Socket programming
- How to C# Chat server programming
- How to C# Chat Server
- How to C# Chat Client
- How to web browser in C#
- No connection could be made because the target machine actively refused it
- System.Net.Sockets.SocketException (0x80004005)
- C# HttpClient - HTTP requests with HttpClient in C#
- C# HttpClient status code