GET, POST, PUT, DELETE Requests | C# HttpClient
C# HttpClient is a class in the .NET framework used for sending HTTP requests and receiving HTTP responses from a resource identified by a URI. It provides a convenient way to make HTTP requests from .NET applications and can be used to perform various operations such as sending GET, POST, PUT, DELETE requests, etc. It's designed to be used for modern, RESTful APIs and supports asynchronous programming.
HTTP request methods
HTTP (Hypertext Transfer Protocol) defines a set of request methods to indicate the desired action to be performed for a given resource. The following are the most common HTTP request methods:
Request | Description |
---|---|
GET | Retrieves data from a specified resource. |
POST | Submits data to be processed to a specified resource. |
PUT | Updates a current resource with new data. |
DELETE | Deletes a specified resource. |
HEAD | Retrieves only the header information from a specified resource. |
CONNECT | Establishes a network connection to a specified resource |
OPTIONS | Describes the communication options for the target resource. |
PATCH | Applies partial modifications to a specified resource. |
These methods are used to indicate the type of operation to be performed on the specified resource and are specified in the request header sent to the server.
C# HttpClient GET request
The GetAsync method is used to send the GET request. The method returns a Task of type HttpResponseMessage, which represents the response from the server.
Follwoing example shows how you can use C# HttpClient to send a GET request:
Explanation:
In the above example, the GetAsync method is used to send the GET request. The method returns a Task of type HttpResponseMessage, which represents the response from the server. The EnsureSuccessStatusCode method is used to check if the response was successful (returned with a status code in the 200-299 range), and if not, it throws an exception. The response content can be read as a string using the ReadAsStringAsync method.
C# HttpClient POST request
The PostAsJsonAsync method is used to send the POST request. The method takes two arguments: the URL to send the request to, and the request content.
The following example shows how you can use C# HttpClient to send a POST request:
Explanation:
In the above example, the PostAsJsonAsync method is used to send the POST request. The method takes two arguments: the URL to send the request to, and the request content. The request content can be any object, which is then serialized to JSON and sent in the request body. The method returns a Task of type HttpResponseMessage, which represents the response from the server. The EnsureSuccessStatusCode method is used to check if the response was successful (returned with a status code in the 200-299 range), and if not, it throws an exception. The response content can be read as a string using the ReadAsStringAsync method.
C# HttpClient PUT Request
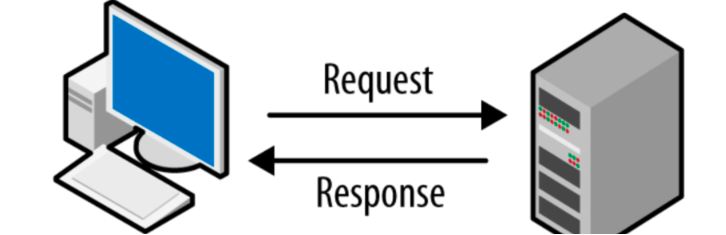
The PutAsJsonAsync method is used to send the PUT request. The method takes two arguments: the URL to send the request to, and the request content.
The following example shows how you can use C# HttpClient to send a PUT request:
Explanation:
In the above example, the PutAsJsonAsync method is used to send the PUT request. The method takes two arguments: the URL to send the request to, and the request content. The request content can be any object, which is then serialized to JSON and sent in the request body. The method returns a Task of type HttpResponseMessage, which represents the response from the server. The EnsureSuccessStatusCode method is used to check if the response was successful (returned with a status code in the 200-299 range), and if not, it throws an exception. The response content can be read as a string using the ReadAsStringAsync method.
C# HttpClient DELETE Request
The DeleteAsync method is used to send the DELETE request. The method takes one argument: the URL to send the request to.
The following example shows how you can use C# HttpClient to send a DELETE request:
Explanation:
In the above example, the DeleteAsync method is used to send the DELETE request. The method takes one argument: the URL to send the request to. The method returns a Task of type HttpResponseMessage, which represents the response from the server. The EnsureSuccessStatusCode method is used to check if the response was successful (returned with a status code in the 200-299 range), and if not, it throws an exception. The response content can be read as a string using the ReadAsStringAsync method.
- How to send email from C#
- How to send email with attachment from C#
- How to send html email from C#
- How to send cdo email from C#
- How to find hostname of a computer
- How to find IP Adress of a computer
- How to read URL Content from webserver
- How to C# Socket programming
- C# Server Socket program
- C# Client Socket program
- C# Multi threaded socket programming
- C# Multi threaded Server Socket programming
- C# Multi threaded Client Socket programming
- How to C# Chat server programming
- How to C# Chat Server
- How to C# Chat Client
- How to web browser in C#
- No connection could be made because the target machine actively refused it
- System.Net.Sockets.SocketException (0x80004005)
- C# HttpClient status code