C# SQL Server Connection
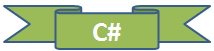
You can connect your C# application to data in a SQL Server database using the .NET Framework Data Provider for SQL Server. The first step in a C# application is to create an instance of the Server object and to establish its connection to an instance of Microsoft SQL Server.
The SqlConnection Object is Handling the part of physical communication between the C# application and the SQL Server Database . An instance of the SqlConnection class in C# is supported the Data Provider for SQL Server Database. The SqlConnection instance takes Connection String as argument and pass the value to the Constructor statement.
Sql Server connection string
If you have a named instance of SQL Server, you'll need to add that as well.
When the connection is established , SQL Commands will execute with the help of the Connection Object and retrieve or manipulate the data in the database. Once the Database activities is over , Connection should be closed and release the Data Source resources .
The Close() method in SqlConnection Class is used to close the Database Connection. The Close method rolls back any pending transactions and releases the Connection from the SQL Server Database.
A Sample C# Program that connect SQL Server using connection string.
Connect via an IP address
1433 is the default port for SQL Server.
Trusted Connection from a CE device
This will only work on a CE device
Connecting to SQL Server using windows authentication
A sample c# program that demonstrate how to execute sql statement and read data from SQL server.
Looking for a C# job ?
There are lot of opportunities from many reputed companies in the world. Chances are you will need to prove that you know how to work with .Net Programming Language. These C# Interview Questions have been designed especially to get you acquainted with the nature of questions you may encounter during your interview for the subject of C# Programming. Here's a comprehensive list of C# Interview Questions, along with some of the best answers. These sample questions are framed by our experts team who trains for .Net training to give you an idea of type of questions which may be asked in interview.
Go to... C# Interview Questions
A sample C# program that perform Data Manipulation tasks like Insert , Update , Delete etc. also perform by the ExecuteNonQuery() of SqlCommand Object.
Full Source C#- C# ADO.NET SqlCommand - ExecuteNonQuery
- C# ADO.NET OleDbCommand - ExecuteNonQuery
- C# ADO.NET SqlCommand - ExecuteScalar
- C# ADO.NET OleDbCommand - ExecuteScalar
- C# ADO.NET SqlCommand - ExecuteReader
- C# ADO.NET OleDbCommand - ExecuteReader
- C# ADO.NET DataReader
- C# ADO.NET SqlDataReader
- C# ADO.NET OleDbDataReader
- C# Multiple Result Sets
- C# Table Schema from SqlDataReader
- C# Table Schema from OleDbDataReader
- C# ADO.NET DataAdapter
- C# ADO.NET SqlDataAdapter
- C# ADO.NET OleDbDataAdapter
- C# ExecuteReader and ExecuteNonQuery
- System.Data.SqlClient.SqlException: Login failed for user