DataGridView Autocomplete TextBox
Presenting data in a tabular format is a recurring task that is frequently encountered in various professional settings. To fulfill this requirement with utmost efficiency, the C# DataGridView control emerges as an indispensable tool, boasting exceptional configurability and extensibility. With its extensive repertoire of properties, methods, and events, this control empowers developers with a wide array of options to carefully tailor both the display and behavior of the tabular representation.
The inherent flexibility of the C# DataGridView control allows for seamless customization to meet specific data presentation needs. Developers have the ability to fine-tune visual attributes such as column widths, cell formatting, and styling, enabling the creation of visually appealing and intuitive table layouts. Additionally, this control's versatility extends to the manipulation of data itself, with comprehensive support for sorting, filtering, and grouping operations, among others, ensuring that the presented information aligns precisely with the intended requirements.
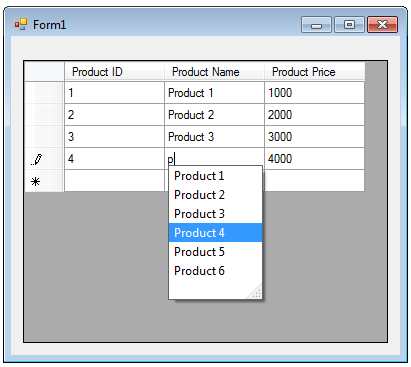
DataGridView

In C# you can display DataGridView in Bound mode, unbound mode and Virtual mode . Bound mode is suitable for managing data using automatic interaction with the data store. The common use of the DataGridView control is binding to a table in a database. The data can be displayed in the DatagridView using TextBox, ComboBox, CheckBox etc. While you display the data on TextBox, you can make your TextBoxes as Autocomplete TextBox.
AutoComplete in DataGridView
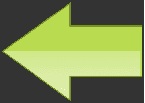
The TextBox control offers a range of properties, such as AutoCompleteCustomSource, AutoCompleteMode, and AutoCompleteSource, that facilitate the automatic completion of user input strings. This feature enhances user convenience by comparing the entered prefix letters to the prefixes of all strings within a designated data source, thereby enabling seamless and efficient input completion.
The following C# program shows how to create an AutoComplete Textbox inside a DataGridView.
Full Source C#Conclusion
The C# DataGridView control stands as a powerful tool for the consistent and professional presentation of data in a tabular format. Its remarkable configurability, extensibility, and comprehensive suite of properties, methods, and events empower developers to effortlessly customize the display and behavior of the control, ensuring that the resulting tabular representation aligns perfectly with the specific requirements of each professional context.
- C# DataGridView Binding - SQL Server dataset
- C# DataGridView Binding - OLEDB dataset
- C# DataGridView Sorting and Filtering
- C# DataGridView Add Columns and Rows
- C# DataGridView Hide Columns and Rows
- C# DataGridView Read Only Columns and Rows
- Add Button to C# DataGridView
- Add CheckBox to C# DataGridView
- Add ComboBox to C# DataGridView
- Add Image to C# DataGridView
- Add ViewLink to C# DataGridView
- C# DataGridView Paging
- C# DataGridView Formatting
- C# DataGridView Template
- C# DataGridView Printing
- C# DataGridView Export to Excel
- C# DataGridView Loading data from Excel
- C# DataGridView Database Operations
- Delete row from datagridview by right click