C# DataGridView Sorting and Filtering
The DataGridView control offers a remarkable degree of configurability and extensibility, presenting a wide range of properties, methods, and events that facilitate effortless customization of its appearance and behavior. A DataView serves as a powerful tool for filtering and sorting data within a DataTable, enabling precise control over data presentation. To illustrate this functionality in action, the following C# program demonstrates the implementation of a DataView to filter and sort a DataGridView, showcasing the seamless integration and utilization of these features.
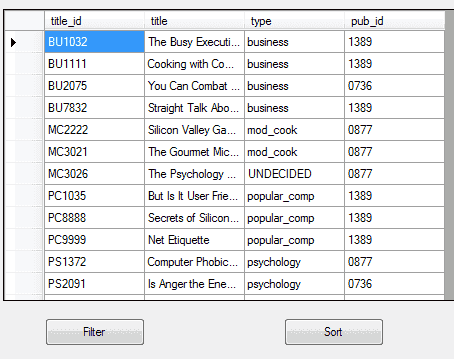
How to sort Datagridview
The DataGridView control in C# offers an inherent feature of automatic sorting, allowing users to effortlessly arrange data within the control by manually sorting any desired column. This versatile functionality empowers users to sort data in either ascending or descending order, based on the contents of the specified column. Additionally, the DataGridView provides a user-friendly experience by visually indicating the sorting operation when a user clicks on the column header. This intuitive behavior ensures a seamless and interactive data sorting experience for users within the DataGridView control.
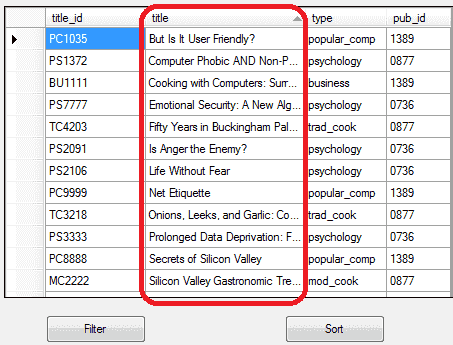
In the above code , datagridview sort the title column thats 1st column.
How to filter Datagridview
There are multiple methods available to filter columns within the DataGridView control, providing users with flexible options to refine and manipulate data. One approach involves sorting the data directly during the retrieval process from the database by utilizing the "order by" clause, ensuring that the desired sorting order is applied right from the source. Alternatively, an alternative method can be employed to accomplish the same objective, as illustrated below.
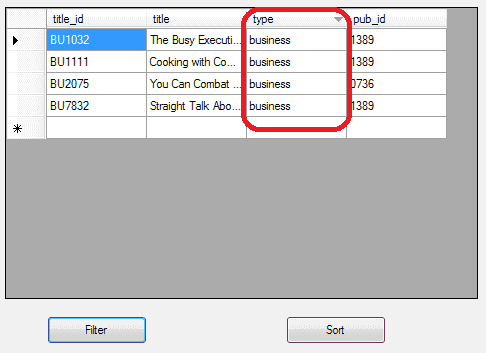
In the above code, datagridview is filter the column Type and value is Business.
Full Source C#- C# DataGridView Binding - SQL Server dataset
- C# DataGridView Binding - OLEDB dataset
- C# DataGridView Add Columns and Rows
- C# DataGridView Hide Columns and Rows
- C# DataGridView Read Only Columns and Rows
- Add Button to C# DataGridView
- Add CheckBox to C# DataGridView
- Add ComboBox to C# DataGridView
- Add Image to C# DataGridView
- Add ViewLink to C# DataGridView
- C# DataGridView Paging
- C# DataGridView Formatting
- C# DataGridView Template
- C# DataGridView Printing
- C# DataGridView Export to Excel
- C# DataGridView Loading data from Excel
- C# DataGridView Database Operations
- Delete row from datagridview by right click
- DataGridView Autocomplete TextBox in C#