Delete row from datagridview by right click
Numerous solutions exist to enable the selection of a row in a DataGridView through right-clicking, subsequently displaying a contextual menu to facilitate deletion. In this particular implementation, we use the CellMouseUp event to handle row selection, while the contextMenuStrip1_Click event is responsible for removing the selected row from the DataGridView.
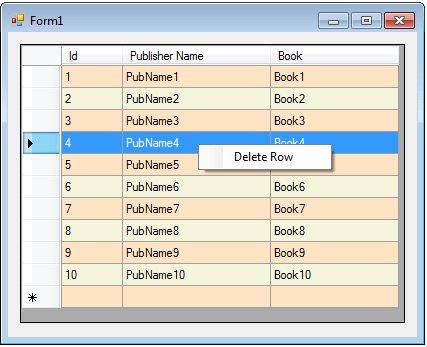
Right click to select row in dataGridView
To begin, it is essential to add a contextMenuStrip control from the toolbox to the designated form. Once added, create a menu item named "Delete Row" within the contextMenuStrip, which will serve as the trigger for row deletion.
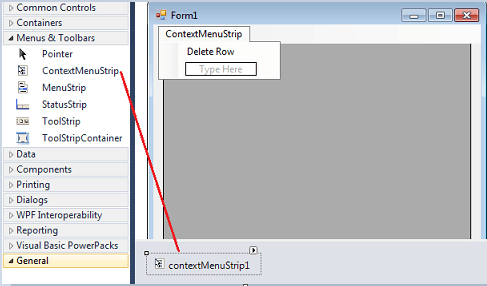
How can I select a row in datagridview on right clcik
Moving forward, the subsequent step involves identifying the appropriate row index upon right-click and displaying the contextMenuStrip accordingly. In this implementation, we utilize the CellMouseUp event of the dataGridView to capture the row index and exhibit the respective menu item. To facilitate future row deletion, a global variable named "rowIndex" is employed to retain the row index value.
Right click and delete row from datagridview
After retrieveing the row index, next step is to delete the specified row. In the contextMenuStrip1_Click event delete the row using rowindex value.
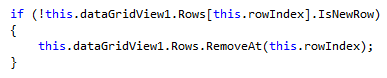
Conclusion
Following this approach, users can seamlessly select rows in the DataGridView through right-clicking, and subsequently trigger the contextMenuStrip to initiate row deletion. This implementation ensures a streamlined and intuitive user experience within the DataGridView.
- C# DataGridView Binding - SQL Server dataset
- C# DataGridView Binding - OLEDB dataset
- C# DataGridView Sorting and Filtering
- C# DataGridView Add Columns and Rows
- C# DataGridView Hide Columns and Rows
- C# DataGridView Read Only Columns and Rows
- Add Button to C# DataGridView
- Add CheckBox to C# DataGridView
- Add ComboBox to C# DataGridView
- Add Image to C# DataGridView
- Add ViewLink to C# DataGridView
- C# DataGridView Paging
- C# DataGridView Formatting
- C# DataGridView Template
- C# DataGridView Printing
- C# DataGridView Export to Excel
- C# DataGridView Loading data from Excel
- C# DataGridView Database Operations
- DataGridView Autocomplete TextBox in C#