Bind a dataset to a combo box in C#
The DataSet is a memory-resident representation of data that offers a consistent relational programming model, irrespective of the data source. It provides a structured way to store and manage data, including tables, relationships, and constraints.
The SqlDataAdapter object allows us to populate DataTables within a DataSet. It facilitates retrieving data from a data source and populating the DataTables using methods like Fill(), providing seamless integration of data into the DataSet.
Bind data source to ComboBox
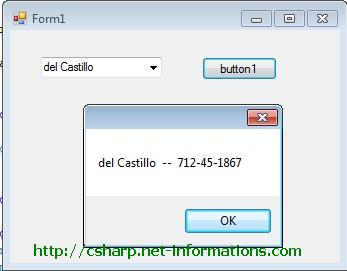
The ComboBox control allows the user to select an option from a list. We can populate a ComboBox from the values in a DataSet, providing a user-friendly interface for selecting options. This is often done by binding the ComboBox to the DataSet's values.
Select Item from ComboBox
The following C# program retrieve the values from database and store it in a dataset and later bind to a combobox.
Binding a ComboBox to an Enum values
Enums in C# provide a convenient way to define a group of related constants that can be represented as strings or integer values. Enums allow for easy selection of options by representing them as a list of string values, which can be bound to a ComboBox for user selection.
Data Binding an Enum with Descriptions
The follwoing C# program bind a combobox with Enum values.
Bind a ComboBox to a generic Dictionary
The Dictionary class in C# is a data structure that represents a collection of key-value pairs. Each key in the dictionary is unique, and it can have at most one associated value. However, a value can be associated with multiple keys. Dictionaries are useful for storing and accessing data based on unique keys.
Dictionary as a Combobox Datasource
The following C# program populating a Combobox from a Dictionary .
Full Source C#- What is C# ADO.NET Dataset
- C# Datset with Sql Server Data Provider
- C# Datset with OLEDB Data Provider
- Find Tables in a Dataset - Sql Server
- Find Tables in a Dataset - OLEDB
- How to Dataset rows count - Sql Server
- How to Dataset rows count - OLEDB
- How to find Column Definition SqlServer
- How to find Column Definition OLEDB
- How to Dyanamic Dataset in C#
- C# Dataset with multiple tables - Sql Server
- C# Dataset with multiple tables - OLEDB
- C# Dataset table relations
- C# Dataset merge tables - Sql Server
- C# Dataset merge tables - OLEDB
- How to find tables in a Database in C#