Deleting Excel WorkSheet using C#
It is possible to delete any worksheet from a Microsoft Excel file programmatically. To achieve this, you need to add a reference to the Microsoft.Office.Interop.Excel assembly in your project. Then, you can utilize classes from that assembly to open the workbook and perform the deletion of the desired worksheet. The program provided below illustrates the process of deleting a worksheet from an existing Excel file using C#.
Excel Library
To access the object model from Visual C# .NET, you have to add the Microsoft Excel 12.0 Object Library to you project. In the previous chapter you can see a step by step instruction on how to add Excel library to your project.
How to add Excel LibraryDelete worksheet from an excel file

In order to delete worksheet from excel file, this program open an existing Excel file and select the worksheet and then delete it.
Delete Excel.Worksheet without prompts
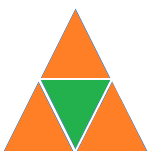
To suppress prompts and alert messages during the execution of a macro in Microsoft Excel, the DisplayAlerts property can be set to False. By doing so, prompts that require user response are effectively suppressed, and Microsoft Excel automatically selects the default response when necessary. Following the completion of the execution process, Microsoft Excel resets the DisplayAlerts property to True, unless the code being executed is cross-process in nature.
It is important to note that modifying the DisplayAlerts property to False ensures a smooth and uninterrupted execution of the macro, as prompts and alert messages that may interrupt the process are suppressed. Once the macro completes its execution, Microsoft Excel reverts the DisplayAlerts property to its default state, allowing for normal prompt behavior, unless the code executed falls within the scope of cross-process operations.
The following source code shows how to programmatically delete Worksheets from Workbooks.
Full Source C#Conclusion
By incorporating these considerations, you can enhance the reliability and seamless operation of your macro by effectively managing prompts and alert messages in Microsoft Excel.
- How to create Excel file in C#
- How to open an Excel file in C#
- How to read an Excel file using C#
- How to programmatically Add New Worksheets
- How to format an Excel file using C#
- How to insert a picture in excel from C# App
- How to insert a background picture in excel
- How to create Excel Chart from C#
- How to export excel chart from C#
- How to excel chart in C# picturebox
- C# data validation input box in excel file
- Read and Import Excel File into DataSet or DataTable
- How to insert data to Excel file using OLEDB
- How to update data in Excel file using OLEDB
- How to export databse to excel file
- How to export DataGridView to excel file