How to create a pdf file in C#
PDF (Portable Document Format) is a versatile file format that faithfully reproduces the characteristics of a printed document, providing a medium that allows for reading, writing, printing, and easy sharing. In C#, you can programmatically generate PDF files with relative ease. When creating documents or graphics in PDF format, they retain their intended appearance as if they were physically printed.
Free PDF Library
PDFSharp is an open-source library that empowers developers to effortlessly create PDF documents directly from their C# applications. With PDFSharp, you can seamlessly generate PDF files, preserving the visual fidelity of the content. Other PDF libraries, such as iTextSharp, are also available on the web to assist with PDF manipulation tasks.
PDFsharp library
PDFsharp is the Open Source .NET library that easily creates and processes PDF documents on the fly from any .NET language. You can freely download the Assemblies version from the following link: Download PDFsharp Assemblies
Steps to create PDF file programmatically.
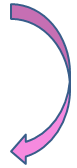
1. Download the Assemblies from the above mentioned url.
2. Extract the .zip file to your desired location (filename :PDFsharp-MigraDocFoundation-Assemblies-1_31.zip)
3. Create a New C# Project
4. Add pdfsharp reference in C# Project
5. In Solution Explorer, right-click the project node and click Add Reference. In this project we are using GDI+ libraries.
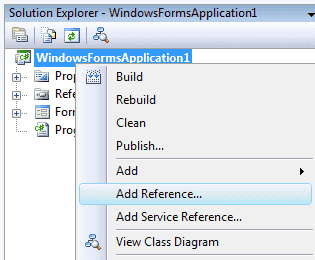
6. In the Add Reference dialog box, select the BROWSE tab and select the Assembly file location (step 2)
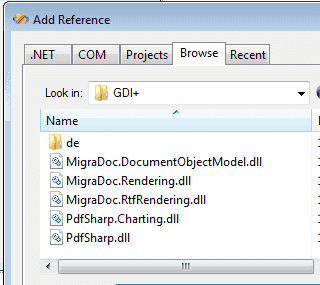
7. Select all files and click OK
After you add the reference files to your C# project , solution explorer look like the following image.
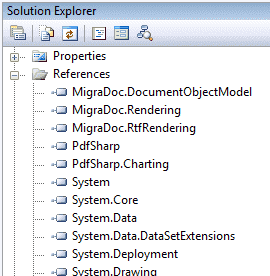
Now you can start programming to create a New PDF document.
First you should create a PDF document Object
Next step is to create a an Empty page.
Then create an XGraphics Object
Also create the Font object from XFont
Next step is that you should write the content to PDF File.
XStringFormats.Center will place the your content to the center of the PDF page.
Now you can save the document as .pdf
You can specify the file path in the pdf.save function.
After save the file , you can double click and open the pdf file. Then you can see the following content in your pdf file.

Drag a Button on the Form and copy and paste the following code in the button1_Click event.
Full Source C#In the above program, a new PDF document is created using the PdfDocument class from PDFsharp. A page is added to the document, and an XGraphics object is obtained for drawing on the page. Text is then drawn on the page using a specified font. Finally, the document is saved as "output.pdf" and closed.
Conclusion
Utilizing libraries like PDFsharp, developers can use the capabilities of the C# language to generate PDF files programmatically, allowing for the creation of professional-looking documents and graphics that can be easily shared and accessed by others.
- How to write a text file in c#
- Append text to an existing file in C#
- How to use C# Directory Class
- How to use C# File Class
- How to use C# FileStream Class
- How to use C# Textreader Class
- A simple C# Text Reader source code
- How to use C# TextWriter Class
- How to use C# BinaryWriter Class
- How to use C# BinaryReader Class
- How to convert XPS file to Bitmap
- How to C# Path Class
- How to create PDF file from Text file
- Write Database data to pdf file
- C# Read Outlook .msg File