Write to Text File in C#
Writing to a text file in C# involves a few simple steps:
- Create a StreamWriter object
- Write to the file
- Close the StreamWriter object
Create a StreamWriter object
To accomplish the task of writing to a text file, it is imperative to commence by creating an instance of the StreamWriter object. This particular class facilitates the availability of robust methods specifically designed for composing content into a text file. In order to instantiate the StreamWriter object, it is necessary to provide explicit instructions encompassing the precise file path and name that will be associated with the created instance.
Following is an example of how to create a StreamWriter object:
Write to the file
After successfully creating the StreamWriter object, you gain access to its assortment of methods, enabling you to accomplish the task of writing content to the file. Among these methods, the Write method holds prominence as the most frequently employed option, as it facilitates the composition of strings directly into the file. Additionally, the WriteLine method presents an alternative solution, allowing you to append strings to the file while automatically including a newline character at the end, thereby facilitating the creation of structured and organized text.
Following is an example of how to write to a file using the StreamWriter object:
Close the StreamWriter object
Once the process of writing to the file has been completed, it is highly advisable to conclude by closing the StreamWriter object, thereby freeing up any resources it may have been utilizing. This crucial step ensures the efficient management of system resources. To accomplish this, simply invoke the Close method on the StreamWriter object, which effectively terminates its association with the file, allowing other processes to access and modify it as needed.
Following is an example of how to close the StreamWriter object:
Write to a text file | C#
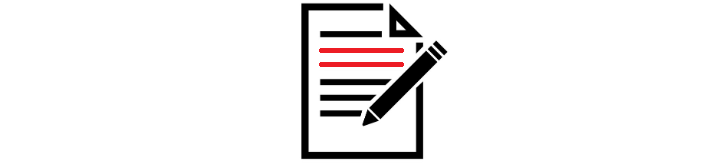
Putting it all together, Following is an example of how to write to a text file in C#:
This will create a file named "file.txt" in the same directory as the program and write the text "Hello, world!" and "This is a new line." to the file.
Write a collection of strings to a file in C#
When there is a need to write a collection of strings to a file, the StreamWriter class proves to be a valuable tool. It facilitates the creation of a new file or the overwriting of an existing file, ensuring that the contents of the collection are accurately reflected within the file.
By utilizing the StreamWriter class, you can efficiently handle the process of writing the collection of strings to the designated file. Whether it involves generating a fresh file or replacing the contents of an existing file, the StreamWriter class offers the necessary functionality to accomplish this task effectively and accurately. Here are the steps to write a collection of strings to a file in C#:
This will create a new file named "file.txt" in the same directory as the program and write each string from the collection on a new line in the file. If the file already exists, it will be overwritten.
- Append text to an existing file in C#
- How to use C# Directory Class
- How to use C# File Class
- How to use C# FileStream Class
- How to use C# Textreader Class
- A simple C# Text Reader source code
- How to use C# TextWriter Class
- How to use C# BinaryWriter Class
- How to use C# BinaryReader Class
- How to convert XPS file to Bitmap
- How to C# Path Class
- How to create a pdf file in C#
- How to create PDF file from Text file
- Write Database data to pdf file
- C# Read Outlook .msg File