How to create PDF file from Text file
Pdfsharp is indeed a widely used open-source framework that enables the programmatic creation of PDF files. In numerous scenarios, PDF documents are preferred over plain text files due to their enhanced capabilities. While text files are considered the simplest file format, limiting users to basic word editing, converting a text file to a PDF document can provide additional functionality and flexibility.
You can freely download the Assemblies version from the following link: Download PDFsharp Assemblies
After download the zip file, extract it and add the reference to your c# project.
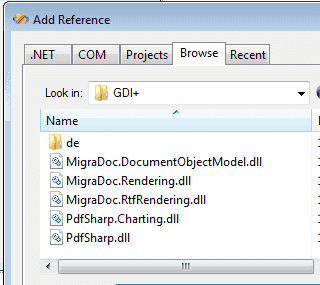
If you want to know the step by step tutorial on how to create your first pdf file programmatically, follow the link : How to create PDF file programmatically
Steps to create PDF file programmatically.
First you need a Text Reader Object to read the text from Text file.
Next step is to create a PDF Object and a Page Object.
Also initialize the Graphics and Font
Now your PDF Object is ready to write the contents, so the next step is to read the content from the Text file and write to the PDF .
In the above code we set X as 40 pixels from the left side and Y as a variable "yPoint", because after write the each line, yPoint will increase 40 pixels line spce then only you get a resonable space between lines.
yPoint = yPoint + 40;
After read all the line from Txt file, you can save the PDF file with your desired name with .pdf extension.
Then close the Reader Object.
Your pdf file look like the following image:
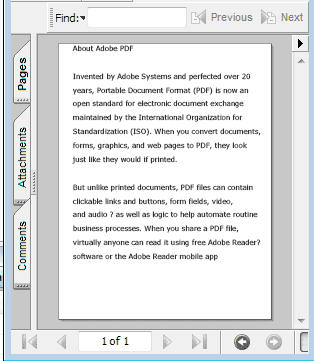
The following program shows how to generate a PDF file from Text file content.
Full Source C#- How to write a text file in c#
- Append text to an existing file in C#
- How to use C# Directory Class
- How to use C# File Class
- How to use C# FileStream Class
- How to use C# Textreader Class
- A simple C# Text Reader source code
- How to use C# TextWriter Class
- How to use C# BinaryWriter Class
- How to use C# BinaryReader Class
- How to convert XPS file to Bitmap
- How to C# Path Class
- How to create a pdf file in C#
- Write Database data to pdf file
- C# Read Outlook .msg File