C# Checked ListBox Control
The CheckedListBox control gives you all the capability of a list box and also allows you to display a check mark next to the items in the list box.
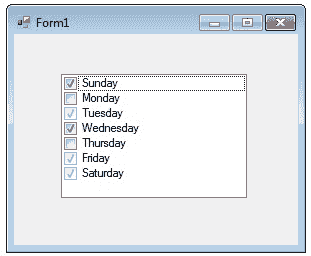
The user can place a check mark by one or more items and the checked items can be navigated with the CheckedListBox.CheckedItemCollection and CheckedListBox.CheckedIndexCollection .
Checkedlistbox add items
SyntaxYou can add individual items to the list with the Add method . The CheckedListBox object supports three states through the CheckState enumeration: Checked, Indeterminate, and Unchecked.
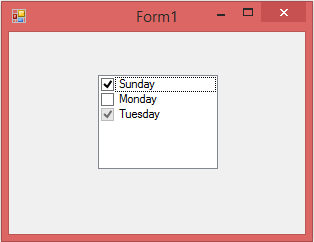
If you want to add objects to the list at run time, assign an array of object references with the AddRange method . The list then displays the default string value for each object.
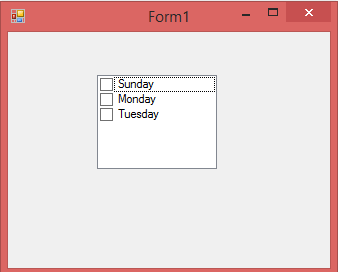
By default checkedlistbox items are unchecked .
Check all items in a Checkedlistbox
If you want to check an item in a Checkedlistbox, you need to call SetItemChecked with the relevant item.
- index(Int32) - The index of the item to set the check state for.
- value(Boolean) - true to set the item as checked; otherwise, false.
If you want to set all items in a CheckedListBox to checked, change the value of SetItemChecked method to true.
Uncheck all items in a Checkedlistbox
If you want to set all items in a CheckedListBox to unchecked, change the value of SetItemChecked method to false.
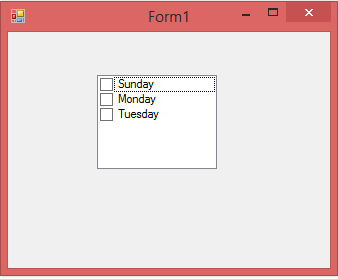
CheckedListBox DataSource
Following example shows how to bind a DataSource to CheckedListBox
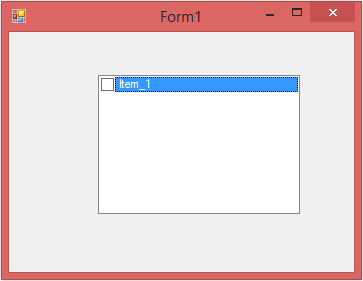
How to get value of checked item from CheckedListBox?
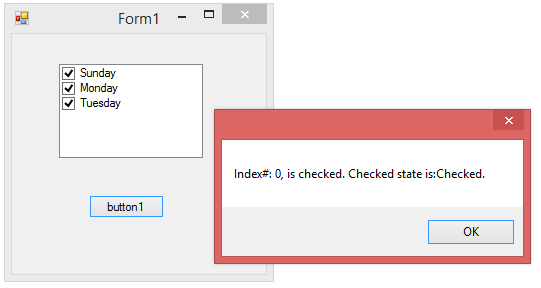
The following example uses the Items property to get the CheckedListBox.ObjectCollection to retrieve the index of an item using the ListBox.ObjectCollection.IndexOf method.
- C# Visual Studio IDE
- How to Create a C# Windows Forms Application
- C# Label Control
- C# Button Control
- C# TextBox Control
- C# ComboBox
- C# ListBox Control
- C# RadioButton Control
- C# CheckBox Control
- C# PictureBox Control
- C# ProgressBar Control
- C# ScrollBars Control
- C# DateTimePicker Control
- C# Treeview Control
- C# ListView Control
- C# Menu Control
- C# MDI Form
- C# Color Dialog Box
- C# Font Dialog Box
- C# OpenFile Dialog Box
- C# Print Dialog Box
- keyPress event in C# , KeyDown event in C# , KeyUp event in C#
- How to create Dynamic Controls in C# ?
- Keep Form on Top of All Other Windows
- C# Timer Control