C# DateTimePicker Control
The DateTimePicker control allows you to display and collect date and time from the user with a specified format.
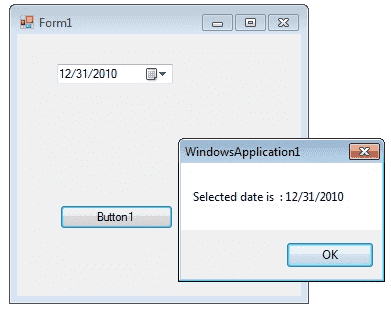
The DateTimePicker control has two parts, a label that displays the selected date and a popup calendar that allows users to select a new date. The most important property of the DateTimePicker is the Value property, which holds the selected date and time.
The Value property contains the current date and time the control is set to. You can use the Text property or the appropriate member of Value to get the date and time value.
The control can display one of several styles, depending on its property values. The values can be displayed in four formats, which are set by the Format property: Long, Short, Time, or Custom.
Convert String to DateTime
You can use the methods like Convert.ToDateTime(String), DateTime.Parse() and DateTime.ParseExact() methods for converting a string-based date to a System.DateTime object. More about..... String to DateTime
How to find date difference ?
The DateTime.Substract method may be used in order to find the date-time difference between two instances of the DateTime method. More about..... Find date difference
How to to set datetime object to null ?
By default DateTime is not nullable because it is a Value Type, using the nullable operator introduced in C# 2, you can achieve this. More about..... Datetime object to null
The following C# program shows how to set and get the value of a DateTimePicker1 control.
Full Source C#- C# Visual Studio IDE
- How to Create a C# Windows Forms Application
- C# Label Control
- C# Button Control
- C# TextBox Control
- C# ComboBox
- C# ListBox Control
- C# Checked ListBox Control
- C# RadioButton Control
- C# CheckBox Control
- C# PictureBox Control
- C# ProgressBar Control
- C# ScrollBars Control
- C# Treeview Control
- C# ListView Control
- C# Menu Control
- C# MDI Form
- C# Color Dialog Box
- C# Font Dialog Box
- C# OpenFile Dialog Box
- C# Print Dialog Box
- keyPress event in C# , KeyDown event in C# , KeyUp event in C#
- How to create Dynamic Controls in C# ?
- Keep Form on Top of All Other Windows
- C# Timer Control