C# ListBox Control
The ListBox control enables you to display a list of items to the user that the user can select by clicking.
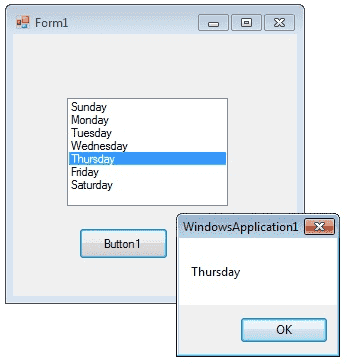
Setting ListBox Properties
You can set ListBox properties by using Properties Window. In order to get Properties window you can Press F4 or by right-clicking on a control to get the "Properties" menu item.
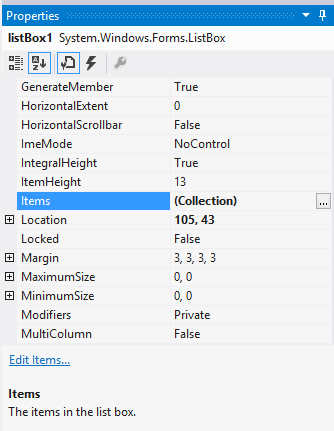
You can set property values in the right side column of the property window.
C# Listbox example
Add Items in a Listbox
SyntaxIn addition to display and selection functionality, the ListBox also provides features that enable you to efficiently add items to the ListBox and to find text within the items of the list. You can use the Add or Insert method to add items to a list box. The Add method adds new items at the end of an unsorted list box.
If the Sorted property of the C# ListBox is set to true, the item is inserted into the list alphabetically. Otherwise, the item is inserted at the end of the ListBox.
Insert Items in a Listbox
SyntaxYou can inserts an item into the list box at the specified index.
Listbox Selected Item
If you want to retrieve a single selected item to a variable , use the following code.
Or you can use
Or
Selecting Multiple Items from Listbox
The SelectionMode property determines how many items in the list can be selected at a time. A ListBox control can provide single or multiple selections using the SelectionMode property . If you change the selection mode property to multiple select , then you will retrieve a collection of items from ListBox1.SelectedItems property.
The ListBox class has two SelectionMode. Multiple or Extended .
In Multiple mode , you can select or deselect any item in a ListBox by clicking it. In Extended mode, you need to hold down the Ctrl key to select additional items or the Shift key to select a range of items.
The following C# program initially fill seven days in a week while in the form load event and set the selection mode property to MultiSimple . At the Button click event it will display the selected items.
Getting All Items from List box
The Items collection of C# Winforms Listbox returns a Collection type of Object so you can use ToString() on each item to display its text value as below:
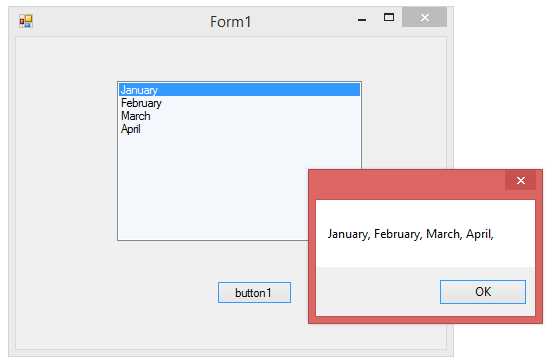
How to bind a ListBox to a List ?
You can bind a List to a ListBox control by create a fresh List Object and add items to the List.
Creating a List
Binding to List
The next step is to bind this List to the Listbox. In order to do that you should set datasource of the Listbox.
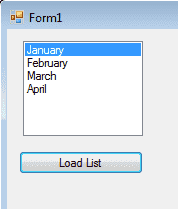
How to bind a listbox to database values ?
First of all, you could create a connection string and fetch data from database to a Dataset.
Set Datasource for ListBox
Next step is that you have set Listbox datasoure as Dataset.
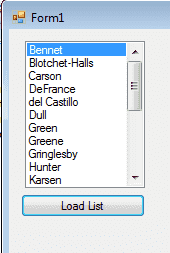
How to refresh DataSource of a ListBox ?
How to clear the Listbox if its already binded with datasource ?When you want to clear the Listbox, if the ListBox already binded with Datasource , you have to set the Datasource of Listbox as null.
How to SelectedIndexChanged event in ListBox ?
This event is fired when the item selection is changed in a ListBox . You can use this event in a situation that you want select an item from your listbox and accodring to this selection you can perform other programming needs.
You can add the event handler using the Properties Window and selecting the Event icon and double-clicking on SelectedIndexChanged as you can see in following image.
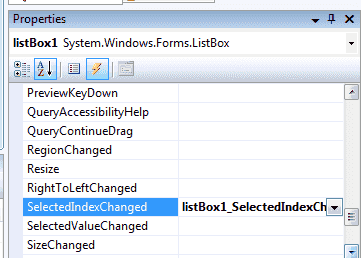
The event will fire again when you select a new item. You can write your code within SelectedIndexChanged event . When you double click on ListBox the code will automatically come in you code editor like the following image.

From the following example you can understand how to fire the SelectedIndexChanged event.
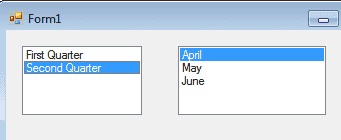
First you should drag two listboxes on your Form. First listbox you should set the List as Datasource , the List contents follows:
When you load this form you can see the listbox is populated with List and displayed first quarter and second quarter. When you click the "Fist Quarter" the next listbox is populated with first quarter months and when you click "Second Quarter" you can see the second listbox is changed to second quarter months. From the following program you can understand how this happened.
C# Listbox Column
A multicolumn ListBox places items into as many columns as are needed to make vertical scrolling unnecessary. The user can use the keyboard to navigate to columns that are not currently visible. First of all, you have Gets or sets a value indicating whether the ListBox supports multiple columns .
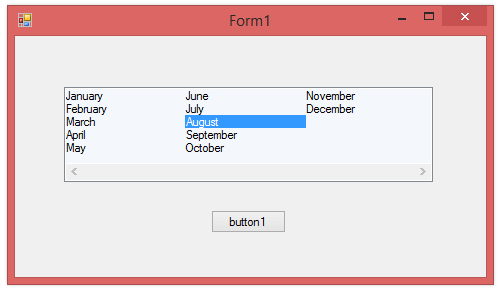
The following C# code example demonstrates a simple muliple column ListBox.
Listbox Item Double Click Event
Add an event handler for the Control.DoubleClick event for your ListBox.
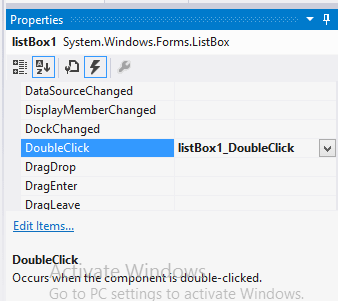
The following program shows when you double click the Listbox Item that event handler open up a MessageBox displaying the selected item.
Listbox vertical scrollbar
ListBox only shows a vertical scroll bar when there are more items then the displaying area. You can fix with ListBox.ScrollAlwaysVisible Property if you want the bar to be visible all the time. If ListBox.ScrollAlwaysVisible Property is true if the vertical scroll bar should always be displayed; otherwise, false. The default is false.
Background and Foreground
The Listbox BackColor and ForeColor properties are used to set the background and foreground colors respectively. If you click on these properties in the Properties window, then the Color Dialog pops up and you can select the color you want.
Alternatively, you can set background and foreground colors from source code. The following code sets the BackColor and ForeColor properties:
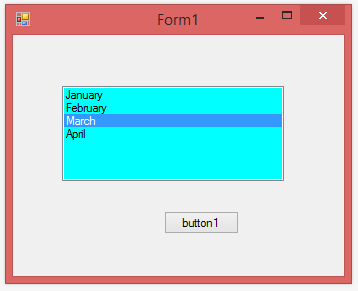
How to clear all data in a listBox?
Listbox Items.Clear() method clear all the items from ListBox.
ListBox.ClearSelected Method Unselects all items in the ListBox.
Remove item from Listbox
RemoveAt method removes the item at the specified index within the collection. When you remove an item from the list, the indexes change for subsequent items in the list. All information about the removed item is deleted.
Listbox Vs ListView Vs GridView
C# ListBox has many similarities with ListView or GridView (they share the parent class ItemsControl), but each control is oriented towards different situations. ListBox is best for general UI composition, particularly when the elements are always intended to be selectable, whereas ListView or GridView are best for data binding scenarios, particularly if virtualization or large data sets are involved. One most important difference is listview uses the extended selection mode by default .
- C# Visual Studio IDE
- How to Create a C# Windows Forms Application
- C# Label Control
- C# Button Control
- C# TextBox Control
- C# ComboBox
- C# Checked ListBox Control
- C# RadioButton Control
- C# CheckBox Control
- C# PictureBox Control
- C# ProgressBar Control
- C# ScrollBars Control
- C# DateTimePicker Control
- C# Treeview Control
- C# ListView Control
- C# Menu Control
- C# MDI Form
- C# Color Dialog Box
- C# Font Dialog Box
- C# OpenFile Dialog Box
- C# Print Dialog Box
- keyPress event in C# , KeyDown event in C# , KeyUp event in C#
- How to create Dynamic Controls in C# ?
- Keep Form on Top of All Other Windows
- C# Timer Control