C# TextBox Control
A TextBox control is used to display, or accept as input, a single line of text. This control has additional functionality that is not found in the standard Windows text box control, including multiline editing and password character masking.
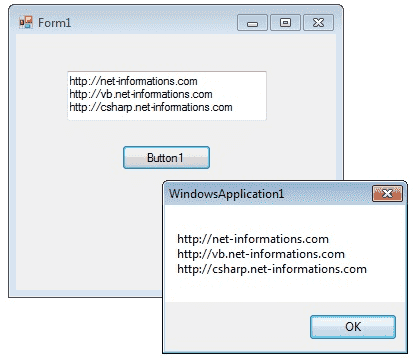
A text box object is used to display text on a form or to get user input while a C# program is running. In a text box, a user can type data or paste it into the control from the clipboard.
For displaying a text in a TextBox control , you can code like this.
You can also collect the input value from a TextBox control to a variable like this way.
C# TextBox Properties
You can set TextBox properties through Property window or through program. You can open Properties window by pressing F4 or right click on a control and select Properties menu item.
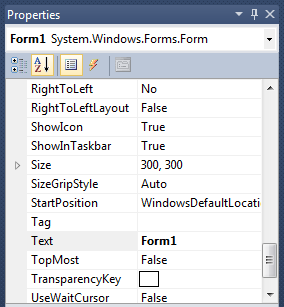
The below code set a textbox width as 250 and height as 50 through source code.
Background Color and Foreground Color
You can set background color and foreground color through property window and programmatically.
Textbox BorderStyle
You can set 3 different types of border style for textbox, they are None, FixedSingle and fixed3d.
TextBox Events
Keydown event
You can capture which key is pressed by the user using KeyDown event
e.g.
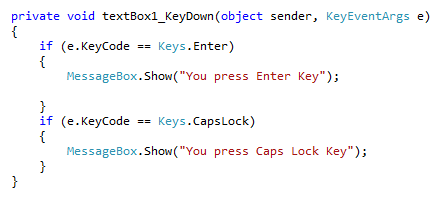
TextChanged Event
When user input or setting the Text property to a new value raises the TextChanged event
e.g.

Textbox Maximum Length
Sets the maximum number of characters or words the user can input into the text box control.
Textbox ReadOnly
When a program wants to prevent a user from changing the text that appears in a text box, the program can set the controls Read-only property is to True.
Multiline TextBox
You can use the Multiline and ScrollBars properties to enable multiple lines of text to be displayed or entered.
Textbox password character
TextBox controls can also be used to accept passwords and other sensitive information. You can use the PasswordChar property to mask characters entered in a single line version of the control
The above code set the PasswordChar to * , so when the user enter password then it display only * instead of typed characters.
How to Newline in a TextBox
You can add new line in a textbox using many ways.
or
How to retrieve integer values from textbox ?
Parse method Converts the string representation of a number to its integer equivalent.
String to Float conversion
String to Double conversion
Looking for a C# job ?
There are lot of opportunities from many reputed companies in the world. Chances are you will need to prove that you know how to work with .Net Programming Language. These C# Interview Questions have been designed especially to get you acquainted with the nature of questions you may encounter during your interview for the subject of .Net Programming. Here's a comprehensive list of .Net Interview Questions, along with some of the best answers. These sample questions are framed by our experts team who trains for .Net training to give you an idea of type of questions which may be asked in interview.
Go to... C# Interview Questions
How to allow only numbers in a textbox
Many of us have faced a situation where we want the user to enter a number in a TextBox. Click the following link that are going to make a Numeric Textbox which will accept only numeric values; if there are any values except numeric. More about.... How do I make a textbox that only accepts numbers
Autocomplete TextBox
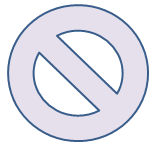
From the latest version of Visual Studio, some of the controls support Autocomplete feature including the TextBox controls. The properties like AutoCompleteCustomSource, AutoCompleteMode and AutoCompleteSource to perform a TextBox that automatically completes user input strings by comparing the prefix letters being entered to the prefixes of all strings in a data source. More about.... C# Autocomplete TextBox
From the following C# source code you can see some important property settings to a TextBox control.
Full Source C#- C# Visual Studio IDE
- How to Create a C# Windows Forms Application
- C# Label Control
- C# Button Control
- C# ComboBox
- C# ListBox Control
- C# Checked ListBox Control
- C# RadioButton Control
- C# CheckBox Control
- C# PictureBox Control
- C# ProgressBar Control
- C# ScrollBars Control
- C# DateTimePicker Control
- C# Treeview Control
- C# ListView Control
- C# Menu Control
- C# MDI Form
- C# Color Dialog Box
- C# Font Dialog Box
- C# OpenFile Dialog Box
- C# Print Dialog Box
- keyPress event in C# , KeyDown event in C# , KeyUp event in C#
- How to create Dynamic Controls in C# ?
- Keep Form on Top of All Other Windows
- C# Timer Control