C# Treeview Control
The TreeView control contains a hierarchy of TreeViewItem controls. It provides a way to display information in a hierarchical structure by using collapsible nodes . The top level in a tree view are root nodes that can be expanded or collapsed if the nodes have child nodes.
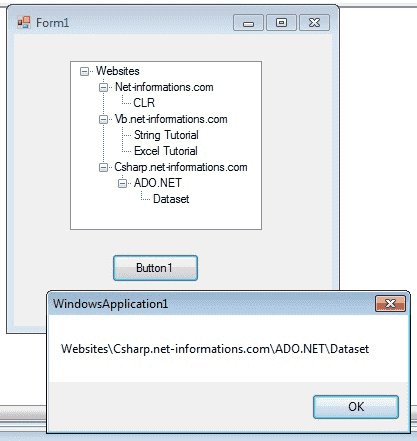
You can explicitly define the TreeView content or a data source can provide the content. The user can expand the TreeNode by clicking the plus sign (+) button, if one is displayed next to the TreeNode, or you can expand the TreeNode by calling the TreeNode.Expand method. You can also navigate through tree views with various properties: FirstNode, LastNode, NextNode, PrevNode, NextVisibleNode, PrevVisibleNode.
The fullpath method of treeview control provides the path from root node to the selected node.
Tree nodes can optionally display check boxes. To display the check boxes, set the CheckBoxes property of the TreeView to true.
The following C# program shows a simple demonstration of treeview control.
Full Source C#- C# Visual Studio IDE
- How to Create a C# Windows Forms Application
- C# Label Control
- C# Button Control
- C# TextBox Control
- C# ComboBox
- C# ListBox Control
- C# Checked ListBox Control
- C# RadioButton Control
- C# CheckBox Control
- C# PictureBox Control
- C# ProgressBar Control
- C# ScrollBars Control
- C# DateTimePicker Control
- C# ListView Control
- C# Menu Control
- C# MDI Form
- C# Color Dialog Box
- C# Font Dialog Box
- C# OpenFile Dialog Box
- C# Print Dialog Box
- keyPress event in C# , KeyDown event in C# , KeyUp event in C#
- How to create Dynamic Controls in C# ?
- Keep Form on Top of All Other Windows
- C# Timer Control