How to create Dynamic Controls in C# ?
How to create Control Arrays in C# ?
Visual Studio .NET does not have control arrays like Visual Basic 6.0 does. The good news is that you can still set things up to do similar things. The advantages of C# dynamic controls is that they can be created in response to how the user interacts with the application. Common controls that are added during run-time are the Button and TextBox controls. But of course, nearly every C# control can be created dynamically.
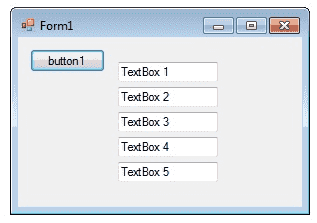
How to create Dynamic Controls in C# ?
The following program shows how to create a dynamic TextBox control in C# and setting the properties dynamically for each TextBox control. Drag a Button control in the form and copy and paste the following source code . Here each Button click the program create a new TextBox control dyanmically.
- C# Visual Studio IDE
- How to Create a C# Windows Forms Application
- C# Label Control
- C# Button Control
- C# TextBox Control
- C# ComboBox
- C# ListBox Control
- C# Checked ListBox Control
- C# RadioButton Control
- C# CheckBox Control
- C# PictureBox Control
- C# ProgressBar Control
- C# ScrollBars Control
- C# DateTimePicker Control
- C# Treeview Control
- C# ListView Control
- C# Menu Control
- C# MDI Form
- C# Color Dialog Box
- C# Font Dialog Box
- C# OpenFile Dialog Box
- C# Print Dialog Box
- keyPress event in C# , KeyDown event in C# , KeyUp event in C#
- Keep Form on Top of All Other Windows
- C# Timer Control