keyPress event in C#
Handle Keyboard Input at the Form Level in C#
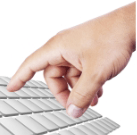
Windows Forms processes keyboard input by raising keyboard events in response to Windows messages. Most Windows Forms applications process keyboard input exclusively by handling the keyboard events.
How do I detect keys pressed in C#
You can detect most physical key presses by handling the KeyDown or KeyUp events. Key events occur in the following order:
- KeyDown
- KeyPress
- KeyUp
How to detect when the Enter Key Pressed in C#
The following C# code behind creates the KeyDown event handler. If the key that is pressed is the Enter key, a MessegeBox will displayed .
How to get TextBox1_KeyDown event in your C# source file ?
Select your TextBox control on your Form and go to Properties window. Select Event icon on the properties window and scroll down and find the KeyDown event from the list and double click the Keydown Event. The you will get the KeyDown event in your source code editor.
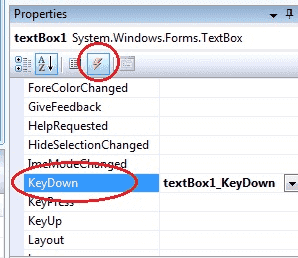
Difference between the KeyDown Event, KeyPress Event and KeyUp Event
KeyDown Event : This event raised as soon as the user presses a key on the keyboard, it repeats while the user keeps the key depressed.
KeyPress Event : This event is raised for character keys while the key is pressed and then released. This event is not raised by noncharacter keys, unlike KeyDown and KeyUp, which are also raised for noncharacter keys
KeyUp Event : This event is raised after the user releases a key on the keyboard.
KeyPress Event :
KeyDown Event :
How to Detecting arrow keys in C#
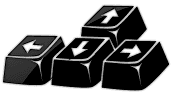
In order to capture keystrokes in a Forms control, you must derive a new class that is based on the class of the control that you want, and you override the ProcessCmdKey().
More about.... Detecting arrow keys from C#
KeyUp Event :
The following C# source code shows how to capture Enter KeyDown event from a TextBox Control.
Full Source C#- C# Visual Studio IDE
- How to Create a C# Windows Forms Application
- C# Label Control
- C# Button Control
- C# TextBox Control
- C# ComboBox
- C# ListBox Control
- C# Checked ListBox Control
- C# RadioButton Control
- C# CheckBox Control
- C# PictureBox Control
- C# ProgressBar Control
- C# ScrollBars Control
- C# DateTimePicker Control
- C# Treeview Control
- C# ListView Control
- C# Menu Control
- C# MDI Form
- C# Color Dialog Box
- C# Font Dialog Box
- C# OpenFile Dialog Box
- C# Print Dialog Box
- How to create Dynamic Controls in C# ?
- Keep Form on Top of All Other Windows
- C# Timer Control