C# Timer Control
What is C# Timer Control ?
The Timer Control plays an important role in the development of programs both Client side and Server side development as well as in Windows Services. With the Timer Control we can raise events at a specific interval of time without the interaction of another thread.
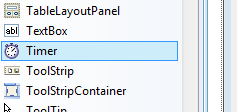
Use of Timer Control
We require Timer Object in many situations on our development environment. We have to use Timer Object when we want to set an interval between events, periodic checking, to start a process at a fixed time schedule, to increase or decrease the speed in an animation graphics with time schedule etc.
A Timer control does not have a visual representation and works as a component in the background.
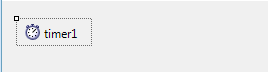
How to use C# Timer Control ?
We can control programs with Timer Control in millisecond , seconds, minutes and even in hours. The Timer Control allows us to set Interval property in milliseconds. That is, one second is equal to 1000 milliseconds. For example, if we want to set an interval of 1 minute we set the value at Interval property as 60000, means 60x1000 .
By default the Enabled property of Timer Control is False. So before running the program we have to set the Enabled property is True , then only the Timer Control starts its function.
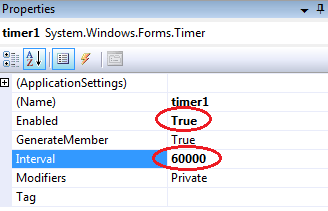
C# Timer example
In the following program we display the current time in a Label Control. In order to develop this program, we need a Timer Control and a Label Control. Here we set the timer interval as 1000 milliseconds, that means one second, for displaying current system time in Label control for the interval of one second.
Start and Stop Timer Control
The Timer control have included the Start and Stop methods for start and stop the Timer control functions. The following C# program shows how to use a timer to write some text to a text file each seconds. The program has two buttons, Start and Stop. The application will write a line to a text file every 1 second once the Start button is clicked. The application stops writing to the text file once the Stop button is clicked.
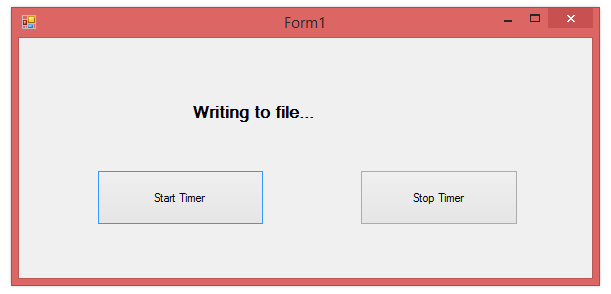
Timer Tick Event
Timer Tick event occurs when the specified timer interval has elapsed and the timer is enabled.
Timer Elapsed Event
Timer Elapsed event occurs when the interval elapses. The Elapsed event is raised if the Enabled property is true and the time interval (in milliseconds) defined by the Interval property elapses.
Timer Interval Property
Timer Interval property gets or sets the time, in milliseconds, before the Tick event is raised relative to the last occurrence of the Tick event .
Timer Reset Property
Timer AutoReset property gets or sets a Boolean indicating whether the Timer should raise the Elapsed event only once (false) or repeatedly (true).
TimerCallback Delegate
Callback represents the method that handles calls from a Timer. This method does not execute in the thread that created the timer; it executes in a separate thread pool thread that is provided by the system.
Timer Class
System.Timers.Timer fires an event at regular intervals . This is a somewhat more powerful timer. Instead of a Tick event, it has the Elapsed event. The Start and Stop methods of System.Timers.Timer which are similar to changing the Enabled property. Unlike the System.Windows.Forms.Timer, the events are effectively queued - the timer doesn't wait for one event to have completed before starting to wait again and then firing off the next event. The class is intended for use as a server-based or service component in a multithreaded environment and it has no user interface and is not visible at runtime.
The following example instantiates a System.Timers.Timer object that fires its Timer.Elapsed event every two seconds sets up an event handler for the event, and starts the timer.
- Create a timer object for one seconds interval.
- Set elapsed event for the timer. This occurs when the interval elapses.
- Finally, start the timer.
C# Timer (Console Application)
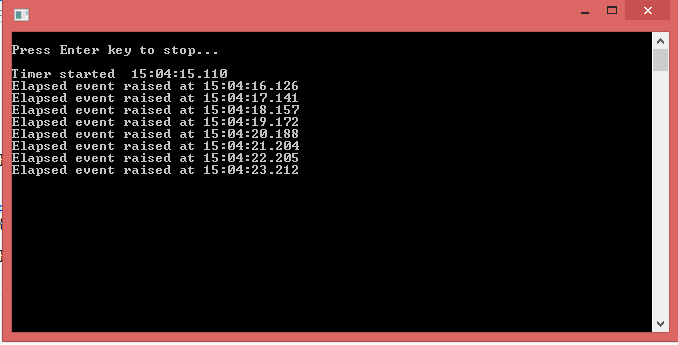
Count down using C# Timer
The following C# program show how to cerate a seconds countdown using C# Timer.
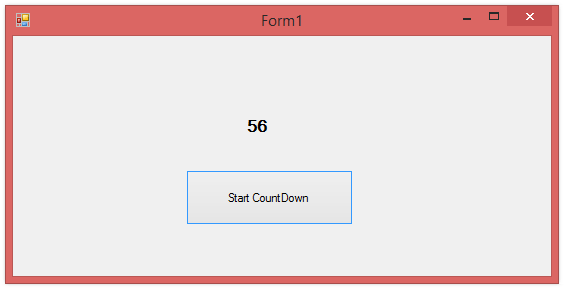
System.Threading.Timer Class
System.Threading.Timer is a simple, lightweight timer that uses callback methods and is served by thread pool threads. It is not recommended for use with Windows Forms, because its callbacks do not occur on the user interface thread. Use a TimerCallback delegate to specify the method you want the Timer to execute. The timer delegate is specified when the timer is constructed, and cannot be changed. The method does not execute on the thread that created the timer; it executes on a ThreadPool thread supplied by the system.
When creating a timer, the application specifies an amount of time to wait before the first invocation of the delegate methods , and an amount of time to wait between subsequent invocations. A timer invokes its methods when its due time elapses, and invokes its methods once per period thereafter. You can change these values, or you can disable the timer, by using the Timer.Change method.
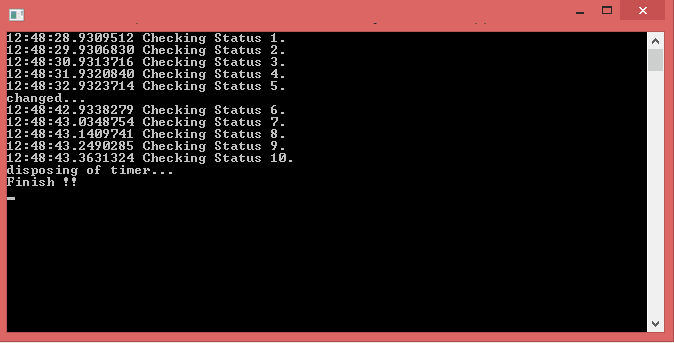
- C# Visual Studio IDE
- How to Create a C# Windows Forms Application
- C# Label Control
- C# Button Control
- C# TextBox Control
- C# ComboBox
- C# ListBox Control
- C# Checked ListBox Control
- C# RadioButton Control
- C# CheckBox Control
- C# PictureBox Control
- C# ProgressBar Control
- C# ScrollBars Control
- C# DateTimePicker Control
- C# Treeview Control
- C# ListView Control
- C# Menu Control
- C# MDI Form
- C# Color Dialog Box
- C# Font Dialog Box
- C# OpenFile Dialog Box
- C# Print Dialog Box
- keyPress event in C# , KeyDown event in C# , KeyUp event in C#
- How to create Dynamic Controls in C# ?
- Keep Form on Top of All Other Windows