System.InvalidCastException
Every value has an associated type in .NET Framework , which defines attributes such as the amount of space allocated to the value, the range of possible values it can have maximum and the members that it makes available .
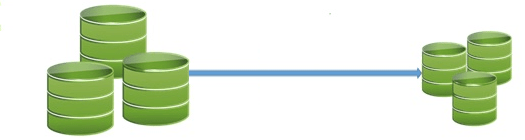
What is Type Conversion?
Type conversion is converting one type of data to another type. This means that, it creates a value in a new type that is equivalent to the value of an old type, but it does not necessarily preserve the exact value (or identity) of the original type. It is also known as Type Casting .
Microsoft .NET Framework automatically supports the following type conversions:- A derived class to a base class.
- A base class back to the original derived class.
- A type that implements an interface to an interface object that represents that interface.
- An interface object back to the original type that implements that interface.
What is InvalidCastException?
An InvalidCastException is thrown when cast from one type to another type is not supported. In some reference type conversions , the compiler cannot determine whether a cast will be valid. It is because of the source type cannot be converted to the destination type , so the cast does not succeed.
For example, if you try to convert a Char value to a DateTime value throws an InvalidCastException exception.
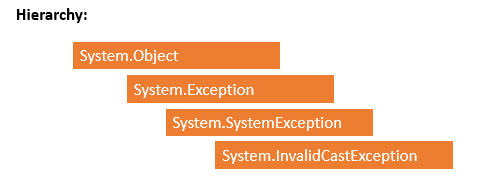
It is important to note that this exception is caused by programming error and should not be handled in a try/catch block; instead, the cause of the exception should be eliminated.
exampleHow to fix InvalidCastException?
System.InvalidCastException is caused by programming error and should not be handled in a try/catch block; instead, the cause of the exception should be eliminated.
is operator
C# provides the "is" operator to enable you to test for compatibility before actually performing a cast operation . It will checks if the runtime type of an expression result is compatible with a given type. The "is operator" returns true if the given object is of the same type otherwise, return false. It also returns false for null objects . It's a straightforward extension of the "is" statement that enables concise type evaluation and conversion.
SyntaxWhere expr is an expression that returns a value and type is the name of a type or a type parameter. The argument expr cannot be an anonymous method or a lambda expression .
exampleToString() method
In the above example, you are trying to convert a value or an object to its string representation by using a casting operator . When you execute the program you will get " System.InvalidCastException : Unable to cast object of type 'System.Int32' to type 'System.String'."
The ToString() method is defined by the Object class and therefore is either inherited or overridden by all managed types. So, here we call its ToString method because it successfully covert an instance of any type to its string representation .

Property | Description |
---|---|
HelpLink | Gets or sets a link to the help file associated with this exception. |
HResult | Gets or sets HRESULT, a coded numerical value that is assigned to a specific exception. |
StackTrace | Gets a string representation of the frames on the call stack at the time the current exception was thrown. |
Method | Description |
---|---|
Equals | Determines whether the specified object is equal to the current object. |
GetType | Gets the runtime type of the current instance. |
ToString | Creates and returns a string representation of the current exception. |
- C# Access Modifiers , CSharp Access Specifiers
- How to CultureInfo in C#
- Difference between readonly and const keyword
- DateTime Format In C#
- Difference Between Task and Thread
- Object reference not set to an instance of an object
- How to Convert Char to String in C#
- How to Convert Char Array to String in C#
- How to convert int to string in C#?