C# Access Modifiers , C# Access Specifiers
Access Modifiers ( Access Specifiers ) describes as the scope of accessibility of an Object and its members. All C# types and type members have an accessibility level . We can control the scope of the member object of a class using access specifiers. We are using access modifiers for providing security of our applications. When we specify the accessibility of a type or member we have to declare it by using any of the access modifiers provided by C# language.
C# provide six access modifiers , they are as follows :
- private
- public
- protected
- internal
- protected internal
- Private Protected (C# version 7.2 and later)
Private Access Modifier
The scope of the accessibility is limited only inside the classes or struct in which they are declared. The private members cannot be accessed outside the class and it is the least permissive access level .
exampleHere we can see the private variable and function in the PrivateAccess class cannot access in the main() function because main() function is in a separated class "Program" . The scope of the accessibility of private access members limited only inside the classes or struct in which they are declared. So, the private members cannot be accessed outside the class and it is the least permissive access level.
exampleHere we made a change to the above program that we move the private variable and function in the same class. So, we can access the private variable and private function from main() because private access modifiers scope is limited only inside the classes or struct in which they are declared.
Public Access Modifier
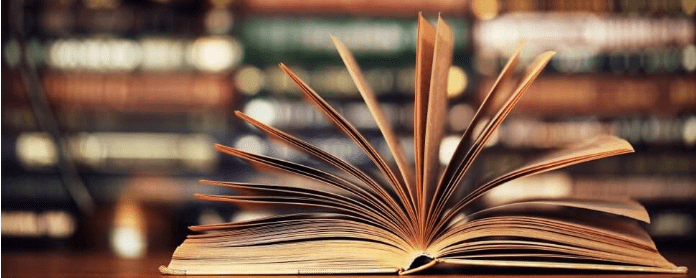
public is the most common access specifier in C# . It can be access from anywhere, that means there is no restriction on accessibility. The scope of the accessibility is inside class as well as outside. The type or member can be accessed by any other code in the same assembly or another assembly that references it.
exampleHere we can access the variable and function in the main() , because the variable and function declared as public.
Protected Access Modifier
The scope of accessibility is limited within the class or struct and the class derived (Inherited )from this class.
example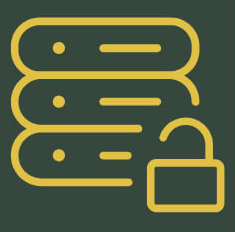
Here we can see the protected variable and function in the ProtectedAccess class cannot access in the main() function because main() function is in a separated class "Program" . The following program will make you clear that how a protected variable and function can access from inherited calss.
exampleHere we made a change to the above program that the class program is in from class "ProtectedAccess" .
So, we can access the protected variable and protected function from main() because Protected access modifiers scope of accessibility is limited within the class or struct and the class derived (Inherited )from this class.
Internal Access Modifier
The internal access modifiers can access within the program that contain its declarations and also access only within files in the same assembly level but not from another assembly.
exampleProtected Internal Access Modifier
Protected internal is the same access levels of both protected and internal . It can access anywhere in the same assembly also be accessed within a derived class in another assembly.
examplePrivate Protected Access Modifier
The private protected access modifier is valid in C# version 7.2 and later .
The protected internal modifier really means protected OR internal, that is - member X is accessible to child classes and also to any class in the current assembly , even if that class is not child of class X (so restriction implied by "protected" is relaxed).
C# version 7.2 and later , the new modifier private protected really means protected AND also internal. That is - member is accessible only to child classes which are in the same assembly, but not to child classes which are outside assembly (so restriction implied by "protected" is narrowed - becomes even more restrictive). That is useful if you build hierarchy of classes in your assembly and do not want any child classes from other assemblies to access certain parts of that hierarchy.
The following example contains two files, Assembly1.cs and Assembly2.cs . The first file contains a public base class, MyBaseClass, and a type derived from it, MyDerivedClass1. MyBaseClass owns a private protected member, count, which MyDerivedClass1 tries to access in two ways. The first attempt to access count through an instance of MyBaseClass will produce an error. However, the attempt to use it as an inherited member in MyDerivedClass1 will succeed. In the second file, an attempt to access count as an inherited member of MyDerivedClass2 will produce an error, as it is only accessible by derived types in Assembly1.
exampleProtected Internal Vs. Private Protected
protected internal
- protected in another assembly: accessible only in the child classes.
- internal in the current assembly: accessible by everyone in the current assembly.
private protected
- private in another assembly: is not accessible.
- protected in the current assembly: accessible only in the child classes.
Default access modifiers in C#
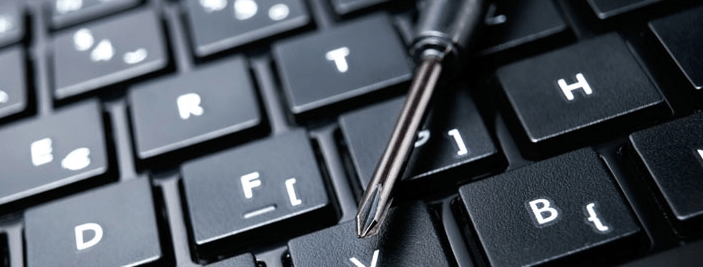
When no access modifier is set, a default access modifier is used. So there is always some form of access modifier even if it's not set. The default access for everything in C# is "the most restricted access you could declare for that member".
- Namespaces implicitly have public declared accessibility. No access modifiers are allowed on C# namespace declarations.
- C# Types declared in compilation units or namespaces can have public or internal declared accessibility and default to internal declared accessibility.
- Class members can have any of the five kinds of declared accessibility and default to private declared accessibility. (It is important to note that a type declared as a member of a class can have any of the five kinds of declared accessibility, whereas a type declared as a member of a namespace can have only public or internal declared accessibility.)
- Struct members can have public, internal, or private declared accessibility and default to private declared accessibility because C# structs are implicitly sealed. Struct members introduced in a struct (that is, not inherited by that struct) cannot have protected or protected internal declared accessibility. (Note that a type declared as a member of a struct can have public, internal, or private declared accessibility, whereas a type declared as a member of a namespace can have only public or internal declared accessibility.)
- Interface members implicitly have public declared accessibility. No access modifiers are allowed on C# interface member declarations.
- C# Enumeration members implicitly have public declared accessibility. No access modifiers are allowed on enumeration member declarations.
Static modifier
The static modifier on a class means that the class cannot be instantiated, and that all of its members are static. A static member has one version regardless of how many instances of its enclosing type are created.
- How to CultureInfo in C#
- How do I solve System.InvalidCastException?
- Difference between readonly and const keyword
- DateTime Format In C#
- Difference Between Task and Thread
- Object reference not set to an instance of an object
- How to Convert Char to String in C#
- How to Convert Char Array to String in C#
- How to convert int to string in C#?