C# DateTime Format
A date and time format string defines the text representation of a DateTime value that results from a formatting operation . C# includes a really great struct for working with dates and time.
You can assign the DateTime object a date and time value returned by a property or method.
Standard date and time format strings
A standard date and time format string in C# uses a single format specifier to define the text representation of a date and time value. A standard or custom format string can be used in two different ways:
- To define the string that results from a formatting operation.
- To define the text representation of a date and time value that can be converted to a DateTime value by a parsing operation.
Following table shows patterns defined in DateTimeFormatInfo and their values for en-US culture.
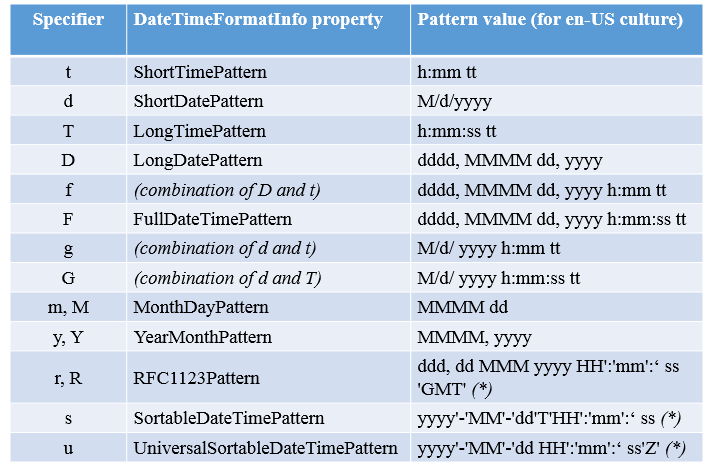
Standard formats are typically used when you need a fast string representation of your DateTime object based on current culture.
C# Standard date and time Example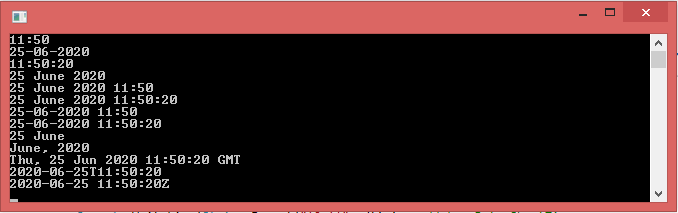
Custom date and time format string
A custom date and time format string consists of two or more characters. Date and time formatting methods interpret any single-character string as a standard date and time format string. In formatting operations , custom date and time format strings can be used either with the ToString method of a date and time instance or with a method that supports composite formatting .
The following table describes various C# DateTime formats .
Format |
---|
DateTime.Now.ToString("MM/dd/yyyy") |
DateTime.Now.ToString("HH:mm") |
DateTime.Now.ToString("hh:mm tt") |
DateTime.Now.ToString("H:mm") |
DateTime.Now.ToString("h:mm tt") |
DateTime.Now.ToString("HH:mm:ss") |
DateTime.Now.ToString("dddd, dd MMMM yyyy") |
DateTime.Now.ToString("dddd, dd MMMM yyyy HH:mm:ss") |
DateTime.Now.ToString("MM/dd/yyyy HH:mm") |
DateTime.Now.ToString("MM/dd/yyyy hh:mm tt") |
DateTime.Now.ToString("MM/dd/yyyy H:mm") |
DateTime.Now.ToString("MM/dd/yyyy h:mm tt") |
DateTime.Now.ToString("MM/dd/yyyy HH:mm:ss") |
DateTime.Now.ToString("MMMM dd") |
DateTime.Now.ToString("yyyy’-‘MM’-‘dd’T’HH’:’mm’:’ss.fffffffK") |
DateTime.Now.ToString("ddd, dd MMM yyy HH’:’mm’:’ss ‘GMT’") |
DateTime.Now.ToString("yyyy’-‘MM’-‘dd’T’HH’:’mm’:’ss") |
DateTime.Now.ToString("yyyy MMMM") |
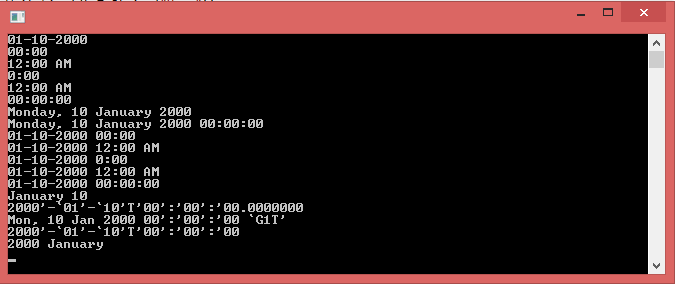
Ticks in DateTime
The Ticks property expresses date and time values in units of one ten-millionth of a second. The Millisecond property returns the thousandths of a second in a date and time value. There are 10,000 ticks in a millisecond, or 10 million ticks in a second. From the following C# example, you can get the milliseconds since 1/1/2000 using such code:
DateTime for a specific culture
CultureInfo provides information about a specific culture . The information includes the names for the culture, the writing system, the calendar used, the sort order of strings, and formatting for dates and numbers.
When you format a DateTime with DateTime.ToString() you can also specify the culture to use.
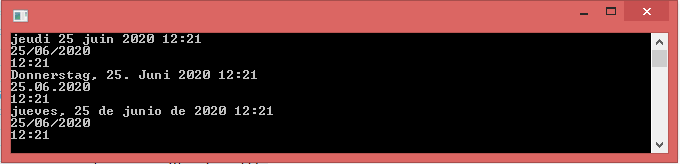
Convert DateTime to String
You can convert a DateTime value to a formatted string like this:
You can convert your string to a DateTime value like this:
Convert String to DateTime
You have basically two options for this.
- DateTime.Parse()
- DateTime.ParseExact()
You can parse user input like this:
If you have a specific format for the string, you should use the other method:
The "d" stands for the short date pattern and null specifies that the current culture should be used for parsing the string .
- C# Access Modifiers , CSharp Access Specifiers
- How to CultureInfo in C#
- How do I solve System.InvalidCastException?
- Difference between readonly and const keyword
- Difference Between Task and Thread
- Object reference not set to an instance of an object
- How to Convert Char to String in C#
- How to Convert Char Array to String in C#
- How to convert int to string in C#?