Object reference not set to an instance of an object
In C#, an object represents an instance of a class and is stored in memory. A reference, on the other hand, serves as a pointer that describes the location in memory where the object is stored. When encountering the message "object reference not set to an instance of an object," it indicates that you are attempting to access or refer to an object that either does not exist, has been deleted, or has been cleaned up. This commonly results in a run-time error, indicating that the program is trying to operate on an object that is not valid or initialized.
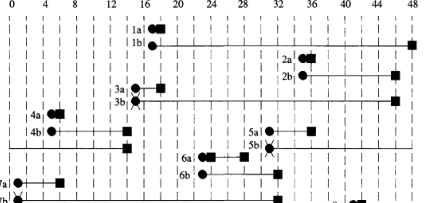
Reference Types
In .NET, variables are categorized as either reference types or value types. Value types encompass primitives like integers and booleans, as well as structures. When declaring value type variables, such as boolean variables, they are automatically assigned a default value:
Reference types, when declared, do not have a default value:
When attempting to access a member of a class, such as in the case of MyClass, if the object reference points to null, it results in a System.NullReferenceException. This exception is synonymous with the message "object reference not set to an instance of an object". It signifies that you are trying to access fields or function types on an object reference that has not been initialized, meaning it is pointing to null. The null-reference exception is intentionally designed as a valid runtime condition, and it can be thrown and caught within the normal flow of a program. Proper handling of this exception is essential for maintaining program stability and preventing unexpected errors.
Handling NullReferenceException
It's usually better to avoid a NullReferenceException than to handle it after it occurs. To prevent the error, objects that could be null should be tested for null before being used.
Object Reference variable is just like "pointer in C" but not exactly a pointer. A NullReferenceException typically reflects developer error and is thrown in the following scenarios:
- Forgotten to instantiate a reference type.
- Forgotten to dimension an array before initializing it.
- Is thrown by a method that is passed null.
- Get a null return value from a method, then call a method on the returned type.
- Using an expression to retrieve a value and, although checking whether the value is null.
- Enumerating the elements of an array that contains reference types, and attempt to process one of the elements.
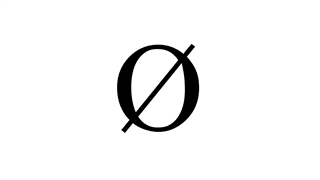
Handling an exception can make your code harder to maintain and understand, and can sometimes introduce other bugs. However, there are many situations where handling the error can be useful:
- Your application can ignore objects that are null.
- You can restore the state of your application to a valid state.
- You want to report the exception.
C#8.0 Nullable reference types
C#8.0 introduces nullable reference types and non-nullable reference types. So only nullable reference types must be checked to avoid a NullReferenceException. Since this is a breaking change, it is launched as an opt-in feature.
- C# Access Modifiers , CSharp Access Specifiers
- How to CultureInfo in C#
- How do I solve System.InvalidCastException?
- Difference between readonly and const keyword
- DateTime Format In C#
- Difference Between Task and Thread
- How to Convert Char to String in C#
- How to Convert Char Array to String in C#
- How to convert int to string in C#?