C# console based application
Console programs indeed offer a straightforward and efficient development experience. Due to their nature of performing input and output solely within the command line interface, console-based applications are particularly useful for swiftly experimenting with language features and developing command-line utilities.
Hello World - Your First Program
The simplicity and directness of console programs make them an ideal starting point for learning programming languages, often exemplified by the classic "Hello, world!" program, which serves as a fundamental introduction to coding concepts and syntax. By crafting a "Hello, world!" program, beginners can quickly gain familiarity with the basic structure and functionality of a programming language. Here also we start with the "Hello, world!".
In order to create a C# console based application
1. Open your Visual Studio
2. On the File menu, click New Project.
Then the New Project dialog box appears. This dialog box lists the different default application types.
3. Select Console Application as your project type and change the name of your application at the bottom textbox.
If you are npt comfortable with default location, you can always enter a new path if you want.
4. Then Click OK.
After click OK button, you will get a screen like the following picture.
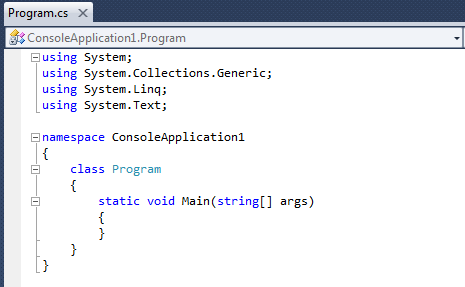
Here in the following program, we just print a "Hello, world!" message only. So copy and paste the following command in the main code block.
Here you can see the full source code.
After enter the command, the next step is to run the program. You can run your program using Ctrl+F5 . Then Visual Studio will keep the console window open, until you press a key. You will get screen look like the following picture.
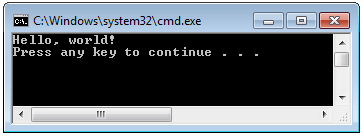
Now you created your first program in Visual Studio.
How to stop C# console applications of closing automatically
When running a console application in Visual Studio, there are two ways to execute it: using Ctrl+F5 or F5.
Using Ctrl+F5 allows the console window to remain open after the program finishes executing, allowing you to view the output and any additional information printed on the console. This method is convenient for programs that don't require debugging or for scenarios where you want the console window to stay open for further interaction.
However, a disadvantage of using Ctrl+F5 is that it bypasses Visual Studio's debug information, making it harder to troubleshoot any issues or bugs within your program.
On the other hand, if you run your console application using F5 (or the "Start Debugging" button), the console window may disappear immediately after the program completes its execution. This behavior is expected as console applications typically close the associated console window once the main method finishes running.
To overcome this and retain the console window for debugging purposes, you can include a read operation at the end of your program, such as using Console.ReadKey(). This will wait for a key press before closing the console window, allowing you to review the output and debug information.
Prevent a C# Console Application from closing when it finishes
To keep the console application open for debugging purposes or to allow the user to view the output, you can use Console.ReadLine() or Console.ReadKey() methods at the end of your program. These methods will wait for user input before proceeding, effectively keeping the application open until the desired input is provided.
Console.ReadLine()
This method reads a line of input from the user, typically terminated by pressing the Enter key. It returns the input as a string, allowing you to capture and process user input as needed. By including Console.ReadLine() at the end of your program, the application will wait for the user to enter something before proceeding further. This is useful for keeping the console window open for inspection or debugging purposes.
Console.ReadKey()
This method reads a single key from the user, allowing you to capture and process the pressed key. It doesn't require the user to press the Enter key, making it suitable for scenarios where you only need to wait for any key press before proceeding. Similar to Console.ReadLine(), including Console.ReadKey() at the end of your program will keep the console window open until a key is pressed.
Full Source:
C# Command Line Parameters
In some situations, you have to send command line parameters to your application. When you pass arguments, the command line input, read from the standard entry point as string array.
The arguments of the Main method is a String array that represents the command-line arguments. Usually you determine whether arguments exist by testing the Length property.
The main program accept arguments in the order of args[0], args[1] etc. The following program shows how to display all parameters enter to the console application.
How to pause console application
System.Threading.Thread.Sleep() will delay your programs. It receives a value indicating the number of milliseconds to wait. This can be useful for waiting on an external application or task.
When you run the above program, it will wait for 5 seconds and exit from application.
How do I correctly exit from a console application
A console application will just terminate when it's done, generally when it runs to the end of its Main entry point method. A "return" will achieve this.
Full Source C#In console-based applications, the program typically exits when the main function has finished executing. However, it's crucial to ensure that any resources you have been managing are properly disposed of to avoid keeping the underlying process alive unnecessarily. In certain situations, you may need to forcefully terminate your program.
To explicitly exit a console application, you can use the Environment.Exit method. This method allows you to immediately terminate the application and close the associated console window.
It's important to exercise caution when using Environment.Exit, as it abruptly terminates the program without allowing for proper cleanup or handling of finalization code. Therefore, it's generally recommended to only use this method when necessary and ensure that any necessary cleanup operations are performed before calling Environment.Exit.