Exception Handling in C#
An exception is an error or unexpected event that occurs while a program is running. Exception handling is an important concept in programming that allows developers to write more robust and error-resistant code.
C# Exceptions (Try..Catch)
Exception handling is a mechanism in C# that allows developers to handle errors that may occur during program execution. When an error occurs in your C# program, an exception is thrown. This exception is a special object that contains information about the error that occurred.
try-catch block
To handle an exception in C#, you can use the try-catch block. The try block contains the code that may throw an exception, while the catch block contains the code that handles the exception if it occurs.
In the above code, ExceptionType is the type of exception that you want to catch. If an exception of this type is thrown within the try block, the code in the catch block will be executed.
The catch block can also specify a parameter (ex in the example above) that represents the exception object that was thrown. This parameter can be used to access information about the exception, such as its message, stack trace, or inner exception.
finally block
C# also provides a finally block, which contains code that is always executed regardless of whether an exception is thrown or not. This block is commonly used for cleanup code, such as releasing resources that were acquired in the try block.
throw statement
In addition to the try-catch-finally block, C# also provides a throw statement, which allows you to manually throw an exception from your program. This can be useful when you want to explicitly indicate that an error has occurred in your code.
In the above code, if someCondition is true, an exception of type Exception will be thrown with the message "An error occurred."
C# Exception Handling Example
Following is a simple example of exception handling in C# that uses a try-catch-finally block to handle exceptions:
Output:
In the above example, the program attempts to divide the integer 10 by 0, which will result in a DivideByZeroException. The code that performs the division is enclosed in a try block, and any exceptions that occur are caught and handled in the catch block. In this case, the catch block simply prints an error message to the console.
The finally block is executed regardless of whether an exception is thrown or not. In this example, the finally block simply prints a message to the console indicating that the program has finished executing.
When you run this program, you'll see that the catch block catches the DivideByZeroException and prints an error message, and the finally block prints a message indicating that the program has finished executing. This example demonstrates how to use try-catch-finally blocks to handle exceptions and ensure that your program executes correctly regardless of any errors that occur.
Exception Hierarchy in C#
The C# Exception Hierarchy is a tree-like structure that represents the inheritance relationships between different types of exceptions in C#. At the root of the hierarchy is the base class System.Exception, from which all other exception types are derived. Here's a brief overview of the hierarchy:
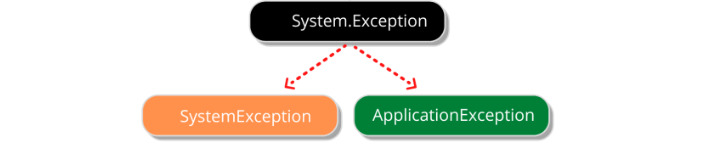
- System.Exception: The base class for all exceptions in C#. It provides properties and methods for working with exceptions.
- System.SystemException: A class that represents system-level exceptions that are thrown by the .NET Framework. Examples of system exceptions include InvalidOperationException, NotImplementedException, and NullReferenceException.
- System.ApplicationException: A class that is intended to be used as a base class for application-level exceptions. It is not used much in modern C# programming.
Following example code to demonstrate how to catch and handle exceptions in the hierarchy:
In the above example, if a DivideByZeroException is thrown, the first catch block will handle it. If a system-level exception is thrown, the second catch block will handle it. Any other type of exception will be caught by the last catch block. By catching and handling exceptions in a way that is specific to the type of error that occurred, you can make your code more robust and reliable.
Using a single catch block for multiple exception types:
You can also use a single catch block that handles multiple exception types by separating the types with the OR ( | ) operator. Here's an example:
In the above example, the catch block handles both DivideByZeroException and ArgumentException exceptions. Any other type of exception will be caught by the last catch block.
User-Defined Exception in C#
In C#, you can create your own user-defined exception types by creating a class that derives from the System.Exception class or one of its derived classes. Here's an example of how to create a custom exception:
In the above example, the MyException class is derived from the Exception class, and it provides three constructors that call the base constructors of the Exception class. This allows you to specify a custom message and inner exception when the exception is thrown.
Once you have defined your custom exception class, you can throw it in your code using the throw keyword. Here's an example:
In the above example, if someCondition is true, the MyMethod method will throw a MyException with a custom message. You can catch and handle this exception just like any other exception in C#. By creating your own custom exceptions, you can provide more specific and meaningful information to the users of your code and make it easier to diagnose and fix errors.
Catching all Exceptions
It is possible to catch all exceptions in C# using a catch block with no exception type specified. This is sometimes referred to as a "catch-all" block.
In the above example, if any exception is thrown in the try block, the catch block will handle it. However, using a catch-all block is generally not recommended because it can make it difficult to diagnose and fix errors. When you catch all exceptions, you may not know what caused the error or how to fix it, which can lead to more problems down the line.
Instead, it's usually better to catch specific exception types that you know how to handle, or to at least catch the base Exception type. By catching specific exception types, you can provide more targeted and meaningful error messages, which can make it easier to diagnose and fix errors.
Re-throwing an Exception in C#
In C#, you can re-throw an exception that has been caught by using the throw keyword inside a catch block. This allows you to pass the exception up the call stack to be handled by higher-level code. Here's an example:
In the above example, if an exception is thrown in the try block, it will be caught by the catch block. The catch block can then handle the exception in any way that is appropriate. Finally, the throw statement inside the catch block re-throws the exception, which allows it to be handled by higher-level code.
When you re-throw an exception, it's important to be careful about how you handle the exception in the higher-level code. If you simply catch the exception again and don't do anything with it, you may end up hiding the original error and making it more difficult to diagnose and fix the problem. On the other hand, if you handle the exception in a meaningful way, such as logging an error message or displaying a user-friendly message, you can make it easier to diagnose and fix the problem.
Summary:
Generally, apart from error handling in C# that comes in handy for dealing with errors and ensuring that your code works as expected even in an unknown environment. Applying the try-catch-finally block and the throw statement enables your program to be highly resilient and reliable with the ability to handle many variances in error scenarios.