How to use C# for loop
C# for loop is a control flow statement that allows you to execute a block of code repeatedly, based on a condition. It is useful for iterating over a fixed set of values or repeating a set of instructions a certain number of times.
Syntax:How C# for loop works:
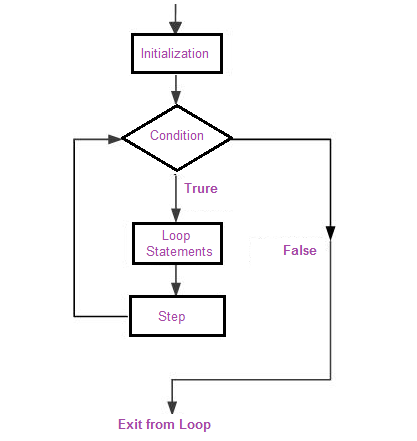
The initialization statement of for loop is executed at the beginning of the loop. This statement initializes the loop control variable to a starting value, and it is typically used to declare the loop control variable and set its initial value.
The condition statement is evaluated at the beginning of each iteration of the loop. If the condition is true, the loop will continue to execute; otherwise, the loop will terminate. The condition typically involves a comparison between the loop control variable and a fixed value or another variable.
The loop body is executed once per iteration, as long as the condition is true. The loop body contains the statements that will be executed repeatedly, and it typically involves manipulating the loop control variable.
The increment statement is executed at the end of each iteration of the loop. This statement modifies the loop control variable in a way that will cause the loop condition to eventually become false. The increment statement is typically used to increment or decrement the loop control variable.
After the increment statement is executed, the condition is evaluated again. If the condition is still true, the loop body is executed again, and the process repeats until the condition becomes false.
C# for loop example:
Following is an example of a C# for loop that iterates from 0 to 4 and prints the value of the loop control variable:
In the above example, the loop control variable "i" is initialized to 0, and the loop will continue to execute as long as "i" is less than 4. The loop control variable is incremented by 1 at the end of each iteration, and the value of "i" is printed to the console using the Console.WriteLine() method.
Output:C# Loop Control Statements | break and continue
C# loop control statements break and continue are used to modify the behavior of loops.
break statement
The break statement is used to immediately terminate the loop, causing control to be transferred to the next statement after the loop. The break statement can be used in any type of loop, including for, while, and do-while loops.
Following is a C# example of using break in a for loop to find the first even number:
In the above example, the for loop iterates from 0 to 4, and on each iteration, it checks whether the current number is even. If it is even, the loop prints the number to the console and immediately terminates using the break statement. As a result, the loop will terminate after finding the first even number (which is 0), and the output will be:
continue statement
The continue statement in for loop is used to skip the current iteration of the loop and move on to the next iteration. The continue statement can also be used in any type of loop.
Following is a C# example of using continue in a for loop to print only odd numbers:
In this example, the for loop iterates from 0 to 5, and on each iteration, it checks whether the current number is odd. If it is odd, the loop skips the current iteration using the continue statement. If it is not odd, the loop prints the number to the console. As a result, the loop will print only odd numbers, and the output will be:
C# Nested for Loop
A nested for loop in C# is a for loop inside another for loop. The outer loop controls the number of iterations, while the inner loop performs a set of iterations on each outer loop iteration.
Following is an example of a nested for loop that prints a multiplication table:
In the above example, the outer loop iterates from 1 to 10, and on each iteration, the inner loop iterates from 1 to 10 as well. On each inner loop iteration, the product of the outer loop index and the inner loop index is printed to the console, separated by spaces. The {0,4} format specifier is used to ensure that each number is printed in a 4-character-wide column.
As a result, the loop will print a multiplication table from 1 to 10, with each row representing a single number from the 1 to 10 range and the columns representing the multiplication of that number with each of the other numbers in the range.
Output:C# Infinite for Loop
An infinite for loop in C# is a loop that continues to execute without end, and it is generally not desirable as it can cause the program to hang or crash.
Following is an example of an infinite for loop:
In the above example, the loop has no initialization, condition, or iterator expressions, resulting in a loop that will continue to execute indefinitely.
How stop an infinite for loop
To stop an infinite for loop, you need to use a loop control statement like break or return to exit the loop at some point. Here's an example of how to use break to exit an infinite loop:
In the above example, the loop will continue to execute indefinitely until the someCondition is met, at which point the break statement is executed to exit the loop.
It's important to avoid creating infinite loops in your programs, as they can consume system resources and cause your program to crash or become unresponsive. It's always a good practice to make sure your loop has a clear exit condition and is not prone to infinite execution.
Multiple Expressions in C# for loop
In C#, a for loop can contain multiple expressions in its header section, separated by commas. These expressions include an initialization expression, a condition expression, and an iterator expression.
Following is an example of a for loop with multiple expressions:
In the above example, the loop has two expressions in the initialization section, where the variable i is initialized to 0, and the variable j is initialized to 10. The loop also has two expressions in the iterator section, where the i variable is incremented by 1, and the j variable is decremented by 1 on each iteration. The loop continues to execute as long as the condition i < j is true.
Output:Multiple expressions in a for loop can be useful when you need to initialize or manipulate multiple variables in the loop header or iterator sections. However, it's important to ensure that these expressions are properly separated and do not conflict with each other to avoid unexpected results.