How to use C# if else statements
The if-else statement in C# allows you to control the flow of your program based on whether a certain condition is true or false.
The basic logic behind an if-else statement is as follows:
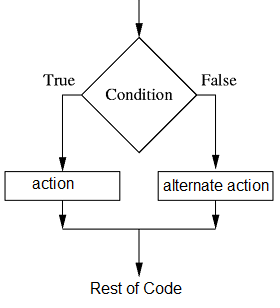
- Evaluate the condition: The condition is evaluated to determine whether it is true or false.
- Execute the appropriate code: If the condition is true, the code inside the first set of curly braces (after the if statement) will be executed. If the condition is false, the code inside the second set of curly braces (after the else statement) will be executed.
C# if statement
The if statement in C# is used to execute a block of code if a given condition is true.
Syntax:Here, the condition is a Boolean expression that returns either true or false. If the condition evaluates to true, the code inside the curly braces { and } will be executed. If the condition evaluates to false, the code inside the curly braces will be skipped.
C# if else statement
In C#, you can also use an if-else statement to execute different blocks of code based on whether a condition is true or false.
Syntax:Here, the code inside the first set of curly braces will be executed if the condition is true, and the code inside the second set of curly braces will be executed if the condition is false.
Multiple if-else statements
You can also chain multiple if-else statements together to create more complex conditionals.
Syntax:In the above example, the first if statement checks condition1. If condition1 is true, the code inside the first set of curly braces will be executed. If condition1 is false, the second if statement checks condition2. If condition2 is true, the code inside the second set of curly braces will be executed. If both condition1 and condition2 are false, the code inside the final set of curly braces will be executed.
Nested if-else statement in C#
In C#, you can also use nested if-else statements, where an if or else statement is contained within another if or else statement. This allows you to create more complex conditions and perform different actions based on multiple criteria.
Syntax:In this example, the first if statement checks condition1. If condition1 is true, the second if statement checks condition2. If both condition1 and condition2 are true, the code inside the first set of nested curly braces will be executed. If condition2 is false, the code inside the second set of nested curly braces will be executed. If condition1 is false, the code inside the final set of curly braces will be executed.
You can nest if-else statements to any depth, allowing you to create complex conditions and take different actions based on multiple criteria. However, it's important to keep the code organized and readable, as nested if-else statements can quickly become difficult to understand.
Conditional Operators in C# if statement
In C#, you can use the conditional OR () and conditional AND ( && ) operators in if statements to combine multiple conditions into a single expression.
Conditional or ( || )
The (conditional OR) operator returns true if either of the conditions is true, and false if both conditions are false. The basic syntax of using the operator in an if statement is as follows:
Syntax:In above example, the condition mark >= 60 attendance >= 75 is evaluated using the conditional OR () operator. If either of the conditions is true, the code inside the first set of curly braces (after the if statement) will be executed and the message "You passed the course." will be printed. If both of the conditions are false, the code inside the second set of curly braces (after the else statement) will be executed and the message "You failed the course." will be printed.
Conditional and (&&)
The && (conditional AND) operator returns true if both conditions are true, and false if either of the conditions is false. The basic syntax of using the && operator in an if statement is as follows:
Syntax:In the above example, the condition mark >= 60 && attendance >= 75 is evaluated using the conditional AND (&&) operator. If both conditions are true, the code inside the first set of curly braces (after the if statement) will be executed and the message "You passed the course." will be printed. If either of the conditions is false, the code inside the second set of curly braces (after the else statement) will be executed and the message "You failed the course." will be printed.
You can use these operators to combine multiple conditions and create more complex expressions in your if statements.
Example:In the above example, the if statement checks whether the person's age is greater than or equal to 18 and whether their gender is either "male" or "female". If both conditions are true, the code inside the curly braces will be executed.