How to use C# switch case statements
The C# switch statement is a control flow statement that allows you to choose from multiple statements based on the value of an expression. It provides a way to execute a specific block of code among several options, depending on the value of the expression.
- expression : An integral or string type expression.
- jump-statement : A jump statement that transfers control out of the case body.
The syntax of the switch statement consists of the keyword switch followed by the expression to be evaluated. Within the body of the switch statement, you define different cases using the case keyword, followed by a constant value or an expression. When the switch statement is executed, the expression is evaluated, and control is transferred to the case that matches the value of the expression.
String Switch
The syntax of the switch statement can be used in C# to achieve string variable comparisons. The switch statement compares the String objects in its expression with the expression phrases related to each case label, using the equals method, which String offers. Having in mind that the switch statement is dependent on the case sensitivity, meaning that it is able to differentiate between the upper or lower case characters. Nonetheless, the usage of the StringComparison preferences for the comparing of string values in the switch statement will also be a good thing. Instead of employing string values in if-else-if chain, the code can be written in a more readable and understandable way by using switch cases. This way eliminates the need for multiple conditions coded which eventually lead into clear and somewhat less verbose code.
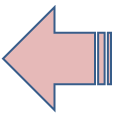
If none of the expressions passed to the switch case match any of the case statements, the control will transfer to the default statement. However, if there is no default statement defined, the control will exit the switch statement and continue with the next set of statements outside of the switch block.
int values with Switch..Case
The following C# program shows how to int values work with Switch..Case statement.
Full Source C#Conclusion
The switch case statements in C# allow for the selection of different code blocks based on the value of an expression. If none of the expressions match any of the case statements, the control can be transferred to a default statement or exit the switch block if there is no default statement defined.