How to use C# while loop
The C# while loop continually executes a block of statements until a specified expression evaluates to false . The expression is evaluated each time the loop is encountered and the evaluation result is true, the loop body statements are executed.
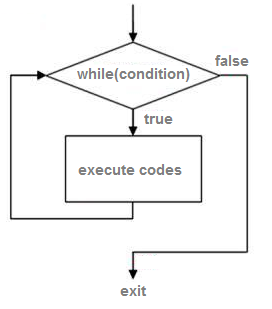
Like if statement the while statement evaluates the expression, which must return a boolean value . If the expression evaluates to true, the while statement executes the statement(s) in the while block . The while statement continues testing the expression and executing its block until the expression evaluates to false.
while loop example
The while loop executes a statement or a block of statements until a specified expression evaluates to false . The above C# while loop example shows the loop will execute the code block 4 times.
How to terminate execution of while loop
A while loop can be terminated when a break , goto , return , or throw statement transfers control outside the loop. To pass control to the next iteration without exiting the loop, use the continue statement.
break statement inside while loop
The break statement provides you with the opportunity to exit out of a while loop when an external condition is triggered. You will put the break statement within the block of code within your loop statement, normally after a conditional if statement. Statements in the loop after the break statement do not execute.
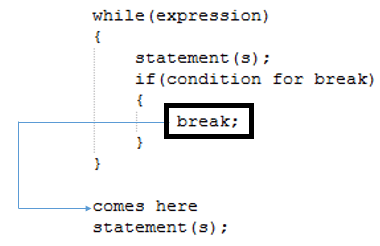
The following program put a condition that when count is greater than 2 then exit from while loop.
When count greater than 2 the program will exit from while loop and goto next statement.
continue statement inside while loop
When a continue statement is encountered inside a while loop, control forces to the beginning of the loop for next iteration , skipping the execution of statements inside the body of loop for the current iteration.
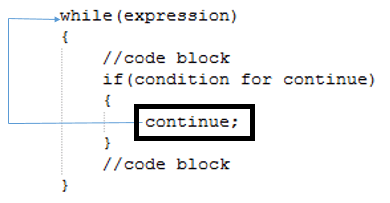
Break Vs Continue
- break - looping is broken and stops.
- continue - loop continues to execute with the next iteration.
goto statement inside while loop
This goto keyword transfers control to a named label
return statement inside while loop
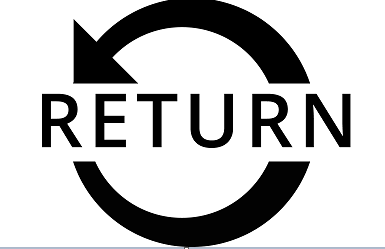
You can return statement, but not recommended because it automatically exits from the current method of execution. Because, it is not useful when other statements after the loop are mandatory.
Multiple condition in while loop
You can use multiple conditions in while loop. You can put multiple conditions using "& &" (AND), "" (OR) in while loop. Both & & and is "short-circuiting" operators, which means that if the answer is known from the left operand , the right operand is not evaluated.
Nested while loop
A loop within another loop is called nested loop.
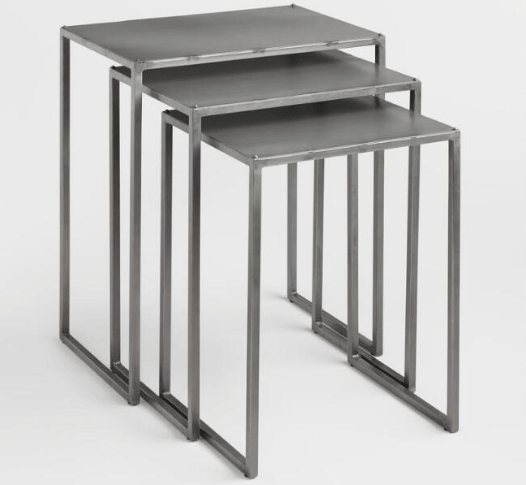
C# while(true) statement
An empty while-loop with this condition is by definition an infinite loop . You can implement an infinite loop using the while statement as follows:
Empty statement
An empty statement is used when you no need to perform an operation where a statement is required. It simply transfers control to the end point of the statement.
Implicitly convert type error
When you run the following program you will get Implicitly convert type error .
In C# while loop , you have to provide a condition(bool) (while(true)) so that the loop continues until the condition is met. You have instead provided an int instead of a condition(bool) , so while(num) is giving you an error. Just change it to while(num!=0) and that should give you a fix.
C# while loop Vs. For loop
Internally, both loops (while and for) compile to the same machine code . The existence of two ways to repeat execution of code stems from two different use cases for each of them.
- For loop knows in advance how many times it will loop, whereas a while loop doesn't know.
- For loop has an initialization step whereas a while loop doesn't.
- For loop uses a "step value" or increment/decrement step, whereas a while loop doesn't.
The while loop iterates through the lines of a document, and processing each line. It is not known in advance how many lines are there. This could not be done easily in a for loop, where you need to know in advance when you are going to stop.
Above is an example of a C# for loop looping from 0 to 99 and printing out the numbers. Notice how the loop is initialized and incremented as part of the for loop structure.
C# while loop Vs. do..while loop
The while loop will check the condition first before executing the content.
The do while loop executes the content of the loop once before checking the condition of the while.
C# while loop programming exercises
- Write a program to display first 10 odd numbers.
Nested while loop examples
- Write a program in to display the pattern like right angle triangle.
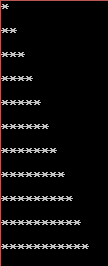
- Write a program to make such a pattern like a pyramid.
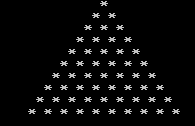
- Write a program to display the pattern like a diamond
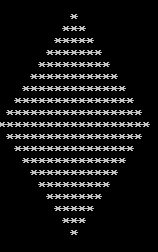