C# Threading Example
Threading is a concept in computer programming that refers to the ability of a program to perform multiple tasks or processes concurrently. In particular, threading refers to the practice of creating threads within a program, which are separate paths of execution that can run concurrently. In C#, threading is supported by the .NET Framework, which provides a set of classes and methods for creating and managing threads.
Create a new thread in C#
To create a new thread in C#, you can either instantiate a new instance of the Thread class and pass it a delegate that represents the code to be executed on the new thread, or you can use the ThreadPool class to queue up work items to be executed on background threads. Here is an example of creating a new thread using the Thread class:
In the above example, the WorkerMethod is executed on a separate thread from the Main method. The Thread.Sleep method is used to simulate some work being done on the worker thread. The Join method is used to wait for the worker thread to complete before continuing on the main thread.
Starting a thread
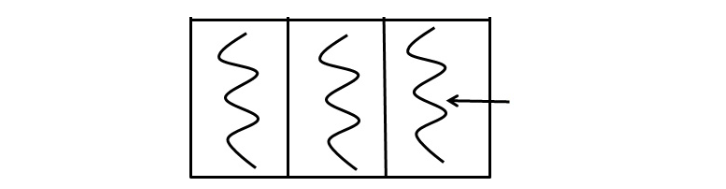
In C#, the Thread.Start method is used to start the execution of a new thread. The Thread.Start method takes no arguments and returns immediately, allowing the calling thread to continue executing.
In the above example, it shows how to use the Thread.Start method to start a new thread:
In the above example, the WorkerMethod is executed on a separate thread from the Main method. The Thread.Start method is called to start the new thread, and then the Main method continues executing.
The Thread.Start method can be used in a variety of scenarios, such as when you need to perform a long-running operation that might block the main thread, or when you need to perform multiple tasks concurrently. It is important to keep in mind that creating and starting new threads can be expensive, so it is generally recommended to use other mechanisms, such as thread pools or async/await, to manage concurrency in your application.
Thread.Join Method (System.Threading)
In C#, the Join method of the Thread class is used to block the calling thread until a specified thread has completed its execution. The Join method can be called on a thread object, and the calling thread will wait for the specified thread to complete before resuming its own execution.
In the above example, it shows how to use the Join method to wait for a worker thread to complete:
In the above example, the WorkerMethod is executed on a separate thread from the Main method. The Join method is used to wait for the worker thread to complete before continuing on the main thread.
When the Join method is called, the calling thread will be blocked until the specified thread completes. If the specified thread has already completed, the Join method will return immediately. If the specified thread is still running, the Join method will block the calling thread until the specified thread has completed.
The Join method is useful when you need to wait for a worker thread to complete before continuing with the rest of the program. It can be used to ensure that all threads have completed their work before the program terminates.
Thread.Sleep Method (System.Threading)
In C#, the Thread.Sleep method is used to pause the execution of the current thread for a specified period of time. The Thread.Sleep method takes a single argument, which specifies the number of milliseconds to pause the thread. During the pause, the thread is blocked and does not consume any CPU time.
In the above example, it shows how to use the Thread.Sleep method to pause the execution of a worker thread:
In the above example, the WorkerMethod is executed on a separate thread from the Main method. The Thread.Sleep method is used to pause the worker thread for 5000 milliseconds (or 5 seconds) before completing.
The Thread.Sleep method can be useful in a variety of scenarios, such as when you need to pause a worker thread to allow other threads to execute, or when you need to add a delay between operations in a time-sensitive application. However, it is important to use the Thread.Sleep method carefully and avoid using it excessively, as it can impact the overall performance and responsiveness of your application.
Conclusion:
C# also provides several synchronization primitives, such as Monitor and Mutex, that can be used to coordinate access to shared resources among multiple threads. It is important to use these synchronization primitives carefully to avoid race conditions and other concurrency issues.