C# thread join method
In C#, the Thread.Join Method (System.Threading) is used to block the calling thread until the thread that it is called on has completed its execution. When the Join method is called, the calling thread will be blocked until the specified thread completes. If the specified thread has already completed, the Join method will return immediately. If the specified thread is still running, the Join method will block the calling thread until the specified thread has completed.
How to use the Thread Join() method in C#
The Join() method is typically used to ensure that the main thread of an application waits for all child threads to complete before exiting, or to synchronize the execution of multiple threads in a complex application.
In the above example, the Main() method creates two new threads, t1 and t2, and starts them using the Start() method. The ThreadWork() method is passed to both threads as the method to execute when the thread is started.
After starting both threads, the main thread calls the Join() method on each thread in turn. This causes the main thread to block until each child thread has completed its execution. Once both child threads have completed, the main thread continues executing and prints a message to the console.
The ThreadWork() method simply prints a message to the console indicating that the thread has started, sleeps for 1 second using the Thread.Sleep() method, and then prints a message indicating that the thread is exiting.
Output:In the above example, the Join() method is used to ensure that the Main() method does not exit until both child threads have completed their work. If the Join() method were not used, it would be possible for the Main() method to exit before the child threads have completed, potentially leaving their work unfinished.
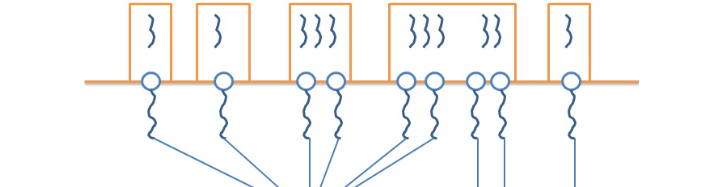
The Join method is useful when you need to wait for a worker thread to complete before continuing with the rest of the program. It can be used to ensure that all threads have completed their work before the program terminates.
In a more complex application, the Join() method might be used in combination with other synchronization mechanisms, such as locks or semaphores, to coordinate the execution of multiple threads and ensure correct operation of the application. For example, in a producer-consumer application where multiple threads are producing and consuming items from a shared queue, the Join() method might be used to ensure that all threads have completed their work before the application shuts down, while locks or semaphores are used to prevent multiple threads from accessing the queue simultaneously and causing race conditions.