How to Terminate a Thread in C#
In C#, there is no direct way to forcefully kill a thread. The Thread.Abort() method can be used to terminate a thread, but it is not recommended because it can leave the application in an inconsistent state. Instead, the preferred way to stop a thread is to use a cooperative approach, where the thread is responsible for checking a shared variable or flag and terminating when requested to do so.
Example:In the above example, the WorkerMethod is executed on a separate thread from the Main method. The while loop in the worker thread will continue running until the Thread.Abort method is called.
The StopThread method is provided to demonstrate how to stop the worker thread by calling the Thread.Abort method. When the Thread.Abort method is called, a ThreadAbortException will be raised on the worker thread. The catch block in the WorkerMethod can be used to handle this exception and perform any necessary cleanup or other actions.
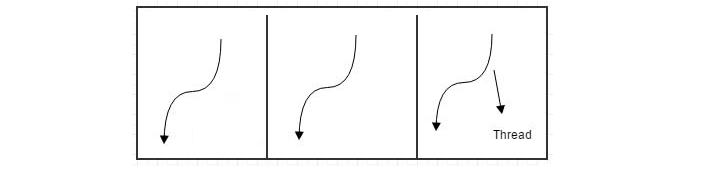
It is important to note that calling Thread.Abort can be dangerous and should be used with caution. If the thread being aborted is holding locks or other resources, those resources may not be released, which can lead to deadlocks or other problems in your application. It is generally recommended to use other mechanisms, such as signaling, to stop threads instead of calling Thread.Abort.