Multithreading in C#
Multithreading in C# refers to the ability to execute multiple threads simultaneously within a single application. This can provide a significant performance boost by allowing the application to utilize multiple CPU cores.
What is a Therad?
A thread in C# is a lightweight and independent unit of execution that enables parallelism and concurrency in software applications. It allows multiple tasks to be executed simultaneously, which can improve application performance and responsiveness. C# provides a rich set of threading APIs that allow developers to create, manage, and synchronize threads with ease. Understanding how to use threads effectively is crucial for building efficient and responsive software applications.
Thread Life Cycle
In C#, a thread is an independent execution path that can run concurrently with other threads. The life cycle of a thread in C# consists of several stages, which are described below:
- Thread creation: In this stage, a new thread is created by calling the Thread class constructor and passing a delegate method or a lambda expression to it. The new thread is not started yet, and it is in the Unstarted state.
- Thread startup: After creating the thread, the Start method is called on the thread object to start the thread. The thread moves from the Unstarted state to the Running state and begins executing the code in the delegate method.
- Thread running: In this stage, the thread is actively executing the code in the delegate method. It will continue to run until it completes its task or is interrupted by another thread or by an exception.
- Thread sleeping: A thread can be made to sleep for a specified period of time by calling the Thread.Sleep method. In this state, the thread is not executing any code and is waiting for the specified time to elapse before resuming execution.
- Thread waiting: A thread can be made to wait for a signal from another thread by calling the WaitOne or WaitAll method on a WaitHandle object. In this state, the thread is not executing any code and is waiting for a signal to be received before resuming execution.
- Thread blocking: A thread can be blocked by calling methods that block the thread until a particular condition is met. For example, the thread can be blocked by calling the Join method on another thread object, which blocks the current thread until the other thread completes.
- Thread aborting: A thread can be aborted by calling the Abort method on the thread object. This causes a ThreadAbortException to be thrown in the thread, which can be caught and handled by the thread's code.
- Thread completing: When a thread completes its task, it moves into the Stopped state. At this point, the thread object can no longer be restarted, and its resources are released.
The life cycle of a thread in C# includes several stages, such as creation, startup, running, sleeping, waiting, blocking, aborting, and completing. Understanding the thread life cycle is essential for writing efficient and robust multithreaded applications.
C# Multithreading
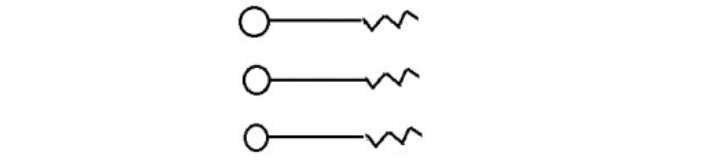
Multithreading is achieved using the System.Threading namespace. This namespace contains classes and interfaces that allow you to create and manage threads in your application.
Creating a Thread
You can create a new thread in C# by instantiating the Thread class and passing it a method to execute:
In the above code, myMethod is the method that will be executed in the new thread.
Synchronization
When multiple threads access shared resources, you need to synchronize their access to avoid conflicts and race conditions. C# provides several synchronization mechanisms to help you do this, including:
- Locks: You can use the lock statement to acquire a mutual-exclusion lock, which ensures that only one thread can access a shared resource at a time.
- Monitor: The Monitor class provides a more advanced synchronization mechanism that allows you to enter and exit critical sections of code.
- Interlocked: The Interlocked class provides atomic operations that allow you to perform simple operations on shared variables without the need for locks.
In the above example, the Worker class has a DoWork method that is executed on multiple threads. The lock statement is used to ensure that only one thread can access the DoWork method at a time. The lockObject is a static object used as a lock to prevent concurrent access to the shared resource. The Thread.Sleep method is used to simulate a time-consuming task.
Thread Synchronization using AutoResetEvent
AutoResetEvent is a synchronization primitive that allows one thread to signal another thread to continue execution. Following an example of how to use AutoResetEvent to synchronize two threads:
Above C# code creates two threads that execute a DoWork() method in the Worker class. The DoWork() method first writes a message to the console, indicating that it is working on a task. Then it sleeps for 1 second (simulating some work being done), writes another message to the console indicating that the task is completed, and finally signals an AutoResetEvent object by calling its Set() method.
The AutoResetEvent object is initially created with a false parameter, meaning that it is in a non-signaled state. The main thread creates an instance of Worker and passes the AutoResetEvent object to it as a parameter. Then, two threads are created, both of which execute the DoWork() method of the Worker object. After each thread has completed its task, it signals the AutoResetEvent object, which then releases one waiting thread (if any).
The Main() method simply waits for user input, which keeps the program running until the user presses a key, at which point the program exits.
A Simple Multithreading Program in C#
Let's take a look at another simple example of how to use multithreading in C#:
In the above example, create two threads t1 and t2. Each thread is assigned a task to perform using the ThreadStart delegate. The Start method is then called on each thread to begin execution. Then call the Join method on each thread to wait for them to complete before continuing with the rest of the program. Finally, print a message to the console indicating that all tasks have completed.
The DoTask1 and DoTask2 methods simply print a message to the console, sleep for a certain amount of time using the Thread.Sleep method, and then print another message to the console indicating that the task has completed.
When you run this program, you will see that both tasks start executing immediately and run simultaneously. Task 2 completes first because it has a shorter sleep time, followed by Task 1. Once both tasks have completed, the program prints the message indicating that all tasks have completed.
Conclusion:
Once you have a good understanding of creating and synchronizing threads in C#, you can explore more advanced topics such as thread pools, async/await, and parallel programming. It's also important to be aware of common issues that can arise in multithreaded code, such as deadlocks and race conditions, and how to avoid or mitigate them.