How to open and read XML file in C#
XML, being a self-describing language, not only holds the data within its tags but also provides the necessary rules and specifications to accurately interpret and extract the information it encompasses. When we engage in reading an XML file, we are essentially accessing and extracting the data that resides within the designated XML tags present in the file.
C# XML Parser
In the previous program, we successfully created an XML file named "products.xml". Now, in the subsequent C# program, we focus on reading the contents enclosed within the XML tags of that file. Reading an XML file offers various approaches, each tailored to specific requirements. In this particular program, we opt for a node-wise content extraction strategy. To accomplish this, we utilize the XmlDataDocument Class, which provides the necessary functionalities for parsing and retrieving data from an XML file. Within this program, the XmlDataDocument Class efficiently navigates through the XML structure, searching for desired nodes and their corresponding child nodes, extracting the pertinent data present within those child nodes.
Click here to download the input file : product.xml
How to read XML file from C#
Reading Xml with XmlReader
The XmlReader is a powerful tool that enables the opening and parsing of XML files in a highly efficient manner. It presents a faster and more memory-friendly alternative to processing XML data. By utilizing the XmlReader, developers can traverse through the XML content step-by-step, examining the values of individual elements, and seamlessly progressing to the subsequent XML elements. This lower-level abstraction offers granular control over the XML file structure, allowing for precise manipulation and retrieval of data.
C# XML Handling
Full Source : Reading Xml with XmlReader
Reading XML with LINQ
You can read Xml file using LINQ also. The following source code shows how to read an XML file using LINQ.
Copy and paste the above XML code to a trext editor and save it as Product.XML
Source Code:
Reading XML with XmlTextReader
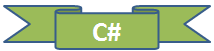
The XmlTextReader is a robust component that facilitates direct parsing and tokenization of XML data, aligning closely with the XML specification outlined by the W3C (World Wide Web Consortium). It adheres to the XML specification standards, including the implementation of namespaces as specified. The XmlTextReader Class serves as a powerful tool, offering forward-only and read-only access to a stream of XML data. This means that it allows sequential traversal of the XML content, enabling efficient and reliable processing of XML information.
Full Source : Reading Xml with XmlReader
Using XPath with XmlDocument
The XmlDocument class plays a crucial role in XML processing by loading the entire XML content into memory, thereby enabling comprehensive navigation and manipulation of the XML data. It provides developers with the flexibility to traverse the XML document both backward and forward, empowering them to efficiently explore the XML structure. Moreover, XmlDocument facilitates advanced querying capabilities through the integration of XPath technology. This powerful combination allows for targeted and precise retrieval of specific elements or data within the XML document, enhancing the overall flexibility and versatility of XML data processing.
Click here to download the input file : product.xml
Full Source C#- How to XML in C#
- How to create an XML file in C#
- How to create XML file from Dataset
- How to Open and read an XML file to Dataset
- How to create an XML file from SQL
- How to search in a XML file
- How to filter in a XML file
- How to insert data from XML to database
- How to create Excel file from XML
- How to create XML file from Excel
- How to XML to DataGridView
- How to create a TreeView from XML File
- How to create a Crystal Reports from XML File
- XML Serialization Tutorial
- XML Serialization
- XML DeSerialization